c# 在一个Person类中,要验证一些字段,其中名称不能为空,出生日期要有效,验证必填项和验证日期 请使用c#中Attribue语法, 并给出具体使用样例
时间: 2024-03-09 17:51:40 浏览: 118
可以在Person类中定义属性时,使用C#中的Attribute语法来进行字段验证。具体步骤如下:
1. 定义一个Attribute类,用于表示要验证的字段信息。例如:
```csharp
public class RequiredAttribute : Attribute { }
public class ValidDateAttribute : Attribute { }
```
2. 在Person类中定义属性时,在属性前面加上定义的Attribute类。例如:
```csharp
public class Person
{
[Required]
public string Name { get; set; }
[ValidDate]
public DateTime BirthDate { get; set; }
}
```
3. 在代码中使用反射来获取属性信息,并根据属性前面的Attribute类进行相应的验证。例如:
```csharp
public static class PersonValidator
{
public static bool Validate(Person person)
{
var properties = typeof(Person).GetProperties();
foreach (var property in properties)
{
var requiredAttribute = property.GetCustomAttribute<RequiredAttribute>();
var validDateAttribute = property.GetCustomAttribute<ValidDateAttribute>();
if (requiredAttribute != null && string.IsNullOrEmpty(property.GetValue(person)?.ToString()))
{
return false;
}
if (validDateAttribute != null && !IsValidDate(property.GetValue(person)))
{
return false;
}
}
return true;
}
private static bool IsValidDate(object value)
{
if (value == null || !DateTime.TryParse(value.ToString(), out var date))
{
return false;
}
return date <= DateTime.Now;
}
}
```
这样,在使用Person类时,可以调用PersonValidator.Validate方法来进行字段验证。例如:
```csharp
var person = new Person
{
Name = "张三",
BirthDate = new DateTime(1990, 1, 1)
};
var isValid = PersonValidator.Validate(person);
```
上述代码中,如果person的Name和BirthDate字段都符合要求,则isValid为true;否则,为false。
阅读全文
相关推荐
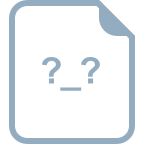
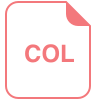
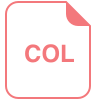
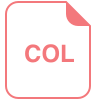
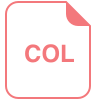
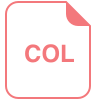
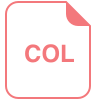
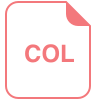
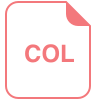
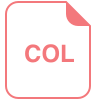
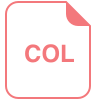
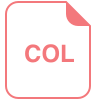
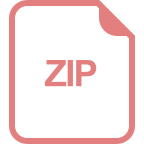
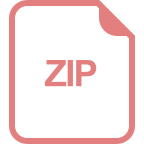
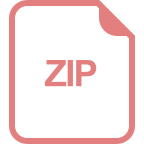
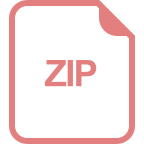
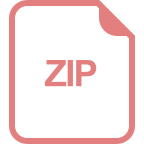
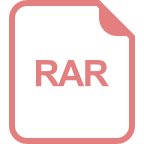