帮我用QT写一个串口插件
时间: 2023-11-15 19:04:14 浏览: 85

QT 之 插件编写
好的,下面是一个简单的串口插件的示例代码,你可以在此基础上进行修改。
1. 创建一个新的QT插件项目
2. 在新项目中添加一个serialport.h文件,用于定义串口接口
```
#ifndef SERIALPORT_H
#define SERIALPORT_H
#include <QObject>
class SerialPort : public QObject
{
Q_OBJECT
public:
explicit SerialPort(QObject *parent = nullptr);
virtual ~SerialPort();
public slots:
virtual void open() = 0;
virtual void close() = 0;
virtual void write(const QByteArray &data) = 0;
signals:
void opened();
void closed();
void error(const QString &errorString);
void dataReceived(const QByteArray &data);
};
#endif // SERIALPORT_H
```
3. 在插件项目中添加一个serialport.cpp文件,实现串口接口
```
#include "serialport.h"
SerialPort::SerialPort(QObject *parent) : QObject(parent)
{
}
SerialPort::~SerialPort()
{
}
```
4. 在插件项目中添加一个serialportplugin.h文件,用于定义插件接口
```
#ifndef SERIALPORTPLUGIN_H
#define SERIALPORTPLUGIN_H
#include <QObject>
#include <QtPlugin>
#include "serialport.h"
class SerialPortPlugin : public QObject
{
Q_OBJECT
public:
explicit SerialPortPlugin(QObject *parent = nullptr);
virtual ~SerialPortPlugin();
virtual SerialPort* createSerialPort(QObject *parent = nullptr) = 0;
};
#define SerialPortPlugin_iid "com.example.SerialPortPlugin"
Q_DECLARE_INTERFACE(SerialPortPlugin, SerialPortPlugin_iid)
#endif // SERIALPORTPLUGIN_H
```
5. 在插件项目中添加一个serialportplugin.cpp文件,实现插件接口
```
#include "serialportplugin.h"
SerialPortPlugin::SerialPortPlugin(QObject *parent) : QObject(parent)
{
}
SerialPortPlugin::~SerialPortPlugin()
{
}
```
6. 在插件项目中添加一个serialportglobal.h文件,用于定义插件的全局变量
```
#ifndef SERIALPORTGLOBAL_H
#define SERIALPORTGLOBAL_H
#include <QtCore/qglobal.h>
#if defined(SERIALPORT_LIBRARY)
# define SERIALPORTSHARED_EXPORT Q_DECL_EXPORT
#else
# define SERIALPORTSHARED_EXPORT Q_DECL_IMPORT
#endif
#endif // SERIALPORTGLOBAL_H
```
7. 在插件项目的.pro文件中添加以下内容
```
TEMPLATE = lib
CONFIG += plugin
QT += core serialport
TARGET = serialport
DESTDIR = $$PWD/bin
HEADERS += \
serialport.h \
serialportplugin.h \
serialportglobal.h
SOURCES += \
serialport.cpp \
serialportplugin.cpp
TARGET = $$qtLibraryTarget(serialport)
```
8. 编译插件项目,生成插件库文件
9. 在使用插件的项目中,添加以下代码来加载插件
```
#include <QtPlugin>
#include "serialportplugin.h"
Q_IMPORT_PLUGIN(SerialPortPlugin)
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
QCoreApplication::addLibraryPath(QCoreApplication::applicationDirPath());
QPluginLoader loader("serialportplugin");
SerialPortPlugin *plugin = qobject_cast<SerialPortPlugin*>(loader.instance());
if (plugin) {
SerialPort *serialPort = plugin->createSerialPort();
if (serialPort) {
serialPort->open();
serialPort->write("Hello World");
serialPort->close();
delete serialPort;
}
}
return a.exec();
}
```
10. 在使用插件的项目中,需要确保插件库文件能够被找到。可以通过将插件库文件放在应用程序的同一目录下,或者使用`QCoreApplication::addLibraryPath`函数添加插件库文件所在的目录。
阅读全文
相关推荐
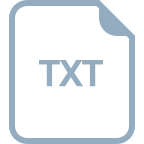
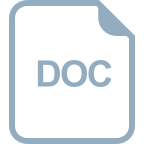

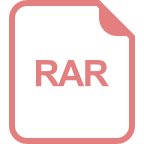
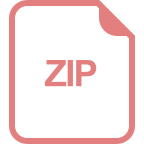
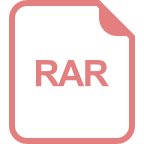
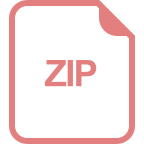
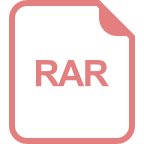
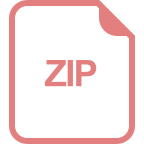
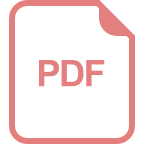
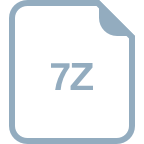
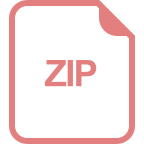
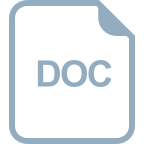
