Once upon a time, Toma found himself in a binary cafe. It is a very popular and unusual place. The cafe offers visitors k different delicious desserts. The desserts are numbered from 0 to k−1 . The cost of the i -th dessert is 2i coins, because it is a binary cafe! Toma is willing to spend no more than n coins on tasting desserts. At the same time, he is not interested in buying any dessert more than once, because one is enough to evaluate the taste. In how many different ways can he buy several desserts (possibly zero) for tasting? Input The first line of the input contains a single integer t (1≤t≤1000 ) — the number of test cases. Then follows t lines, each of which describes one test case. Each test case is given on a single line and consists of two integers n and k (1≤n,k≤109 ) — the number of coins Toma is willing to spend and the number of desserts in the binary cafe. Output Output t integers, the i -th of which should be equal to the answer for the i -th test case — the number of ways to buy desserts for tasting.
时间: 2024-02-14 07:11:02 浏览: 138
This is a classic problem of finding the number of subsets of a given set with a certain property. In this case, we want to find the number of subsets of the set {0,1,2,...,k-1} such that the sum of the elements in the subset is less than or equal to n.
We can use dynamic programming to solve this problem efficiently. Let dp[i][j] be the number of ways to choose a subset of the first i elements of the set {0,1,2,...,k-1} such that the sum of the elements in the subset is exactly j. Then the answer to the problem is the sum of dp[k][0] to dp[k][n].
The base case is dp[0][0]=1, since there is exactly one way to choose an empty subset with sum zero. For each i from 1 to k, we can either choose the i-th element or not. If we choose it, then we need to find the number of ways to choose a subset of the first i-1 elements with sum j-2i. If we do not choose it, then we need to find the number of ways to choose a subset of the first i-1 elements with sum j. Therefore,
dp[i][j] = dp[i-1][j] + dp[i-1][j-2i], if j>=2i
dp[i][j] = dp[i-1][j], otherwise
The final answer is the sum of dp[k][0] to dp[k][n]. The time complexity of this algorithm is O(kn).
Here is the Python code to solve the problem:
```python
t = int(input())
for _ in range(t):
n, k = map(int, input().split())
dp = [[0] * (n+1) for _ in range(k+1)]
dp[0][0] = 1
for i in range(1, k+1):
for j in range(n+1):
dp[i][j] = dp[i-1][j]
if j >= 2**i:
dp[i][j] += dp[i-1][j-2**i]
print(sum(dp[k]))
```
I hope this helps! Let me know if you have any more questions.
阅读全文
相关推荐
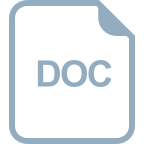
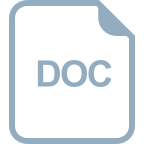
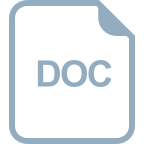


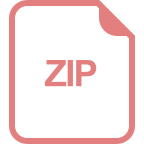
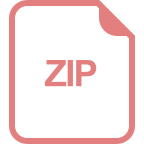
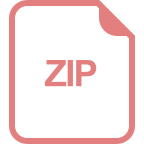
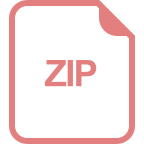
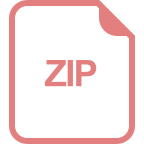
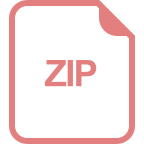
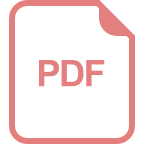
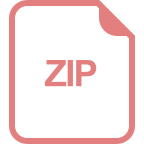
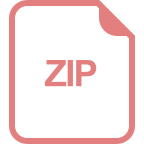
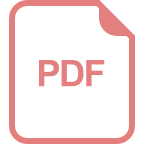
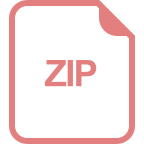
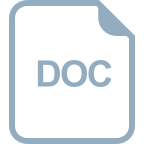
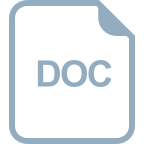