基于c语言的交通灯程序设计
时间: 2023-07-13 21:20:52 浏览: 84
以下是一个基于C语言的简单交通灯程序设计示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main()
{
int red = 1, yellow = 0, green = 0; // 初始化红、黄、绿三个灯的状态为红灯
int timeCount = 0; // 定义计时器
srand((unsigned)time(NULL)); // 初始化随机数种子
while (1) // 循环执行交通灯程序
{
// 输出当前状态的交通灯
printf("Red Light: %s\n", red ? "On" : "Off");
printf("Yellow Light: %s\n", yellow ? "On" : "Off");
printf("Green Light: %s\n", green ? "On" : "Off");
// 红灯状态下计时10秒
if (red)
{
timeCount++;
if (timeCount >= 10)
{
timeCount = 0;
red = 0;
green = 1;
}
}
// 绿灯状态下计时20秒
else if (green)
{
timeCount++;
if (timeCount >= 20)
{
timeCount = 0;
green = 0;
yellow = 1;
}
}
// 黄灯状态下计时5秒
else if (yellow)
{
timeCount++;
if (timeCount >= 5)
{
timeCount = 0;
yellow = 0;
red = 1;
}
}
// 在每个状态结束前随机停留1-3秒
int delay = rand() % 3 + 1;
printf("Delay for %d seconds...\n\n", delay);
sleep(delay);
}
return 0;
}
```
这个程序实现了红、黄、绿三个灯的状态切换,每个状态的持续时间分别为10秒、20秒和5秒,同时每个状态结束前会随机停留1-3秒。可以根据实际需求修改计时器和延时时间的值。
阅读全文
相关推荐
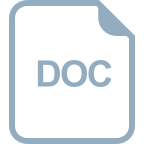
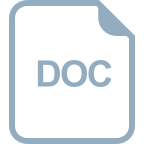
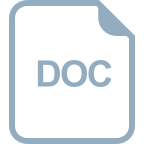
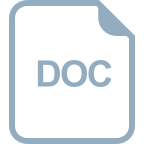
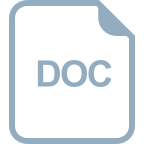
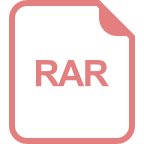
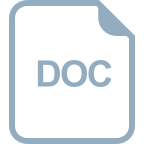
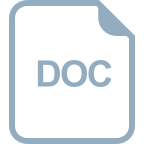
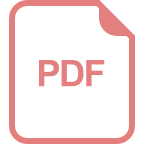
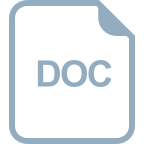
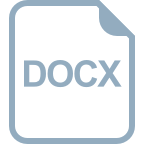
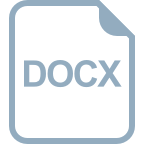
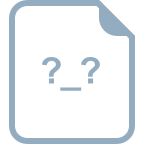
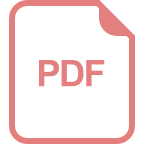

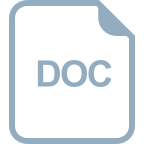
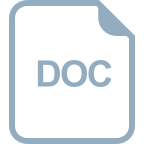
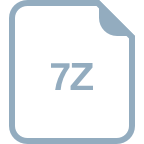