python统计字符串中子串出现的次数
时间: 2023-06-05 17:47:31 浏览: 204
可以使用Python中的字符串函数count()来统计子串在字符串中出现的次数。例如:
```python
s = 'python练习字符串中子串出现的次数'
sub = '字符串'
count = s.count(sub)
print(count) # 输出2
```
以上代码中,字符串s为要统计的字符串,子串sub为要计算出现次数的子串。使用count()函数即可返回子串在字符串中出现的次数。
相关问题
如何统计字符串中子字符串出现的次数,不论大小写
可以通过将字符串和子字符串都转换为小写或大写来实现不区分大小写的统计。以下是一个示例代码:
```python
def count_substring(string, sub):
# 将字符串和子字符串都转换为小写
string = string.lower()
sub = sub.lower()
# 使用 count() 统计子字符串出现的次数
return string.count(sub)
```
使用示例:
```python
s = "Hello, hello, how are you today?"
sub = "hello"
count = count_substring(s, sub)
print(count) # 输出:2
```
注意,这里使用了字符串的 `lower()` 方法将字符串转换为小写。如果需要统计大写字母出现次数,可以将 `lower()` 改为 `upper()`。
统计字符串中子串出现的次数
以下是两种统计字符串中子串出现次数的方法:
1.使用count()函数
```python
str = "hello world, world is beautiful"
substr = "world"
count = str.count(substr)
print("子串出现的次数为:", count) # 输出:子串出现的次数为: 2
```
2.使用循环逐个比较
```python
str = "hello world, world is beautiful"
substr = "world"
count = 0
for i in range(len(str)-len(substr)+1):
if str[i:i+len(substr)] == substr:
count += 1
print("子串出现的次数为:", count) # 输出:子串出现的次数为: 2
```
阅读全文
相关推荐
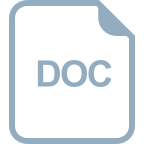
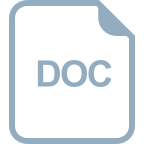
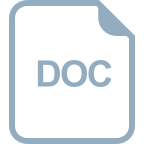








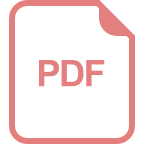

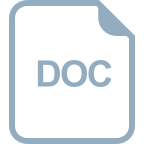
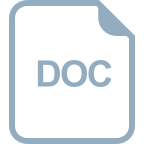
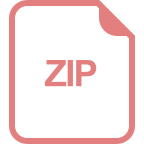