QString 的正则表达式用法
时间: 2023-11-10 14:57:53 浏览: 153
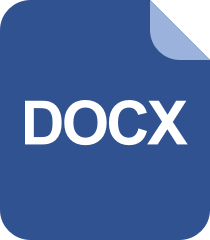
QT中QString类中文详解
QString 类提供了一系列正则表达式的方法,可以用来匹配、替换、捕获等操作。以下是一些常见的用法:
1. 字符串匹配
```cpp
QString str = "hello world";
QRegExp regex("world");
if (regex.indexIn(str) != -1) {
qDebug() << "Matched";
} else {
qDebug() << "Not matched";
}
```
2. 捕获子串
```cpp
QString str = "hello world";
QRegExp regex("(.*) world");
if (regex.indexIn(str) != -1) {
qDebug() << "Matched";
qDebug() << regex.cap(1); // 输出 "hello"
} else {
qDebug() << "Not matched";
}
```
3. 替换字符串
```cpp
QString str = "hello world";
QRegExp regex("world");
str.replace(regex, "Qt");
qDebug() << str; // 输出 "hello Qt"
```
4. 判断字符串是否符合规则
```cpp
QString str = "password123";
QRegExp regex("^[a-zA-Z]\\w{5,17}$"); // 匹配以字母开头,长度为6-18的字符串
if (regex.exactMatch(str)) {
qDebug() << "Valid password";
} else {
qDebug() << "Invalid password";
}
```
5. 分割字符串
```cpp
QString str = "hello,world,Qt";
QStringList list = str.split(QRegExp("[,]"));
qDebug() << list; // 输出 ["hello", "world", "Qt"]
```
以上只是一些常见用法,Qt 的正则表达式支持更多的功能,可以参考文档进行学习和使用。
阅读全文
相关推荐
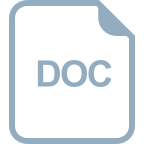
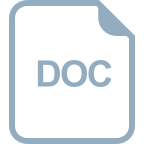



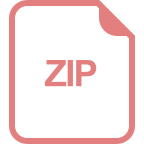











