html实现用户点击‘确定’按钮,页面显示“正在分析,请稍后”,等待5秒后跳转至resul.html页面
时间: 2023-11-27 18:03:23 浏览: 583
可以使用以下HTML代码实现:
```html
<!DOCTYPE html>
<html>
<head>
<title>等待跳转页面</title>
<script>
function showText() {
document.getElementById("status").innerHTML = "正在分析,请稍后";
setTimeout(redirect, 5000);
}
function redirect() {
window.location.href = "result.html";
}
</script>
</head>
<body>
<button onclick="showText()">确定</button>
<p id="status"></p>
</body>
</html>
```
当用户点击“确定”按钮时,会触发`showText()`函数,该函数会将`<p>`标签的内容修改为“正在分析,请稍后”,并在5秒后调用`redirect()`函数进行页面跳转。`redirect()`函数中使用`window.location.href`将页面跳转至`result.html`页面。
相关问题
Django相关实现:views中resul函数会从数据库test表最后一行中得到一个视频的名称与所处位置,然后会将这两个属性传给result.html,result.html显示的页面能够播放该视频
实现这个功能,你需要以下步骤:
1. 在views.py中,导入test模型和HttpResponse:
```python
from django.http import HttpResponse
from .models import test
```
2. 创建result函数,从test表中获取最后一行数据:
```python
def result(request):
# 从test表中获取最后一行数据
data = test.objects.last()
# 获取视频名称和位置
video_name = data.video_name
video_location = data.video_location
# 将数据传递给result.html
return render(request, 'result.html', {'video_name': video_name, 'video_location': video_location})
```
3. 在result.html中,使用video标签来播放视频:
```html
<video src="{{ video_location }}" controls>
Your browser does not support the video tag.
</video>
```
其中,`{{ video_location }}`是从视图函数中传递过来的视频位置。
4. 将urls.py中的url映射到result函数:
```python
from django.urls import path
from . import views
urlpatterns = [
path('result/', views.result, name='result'),
]
```
现在,当你访问`/result/`页面时,就会自动播放test表中最后一行数据所指定的视频。
在当前目录下有一个data.bxt文件,里面有若干行,每行的示下: 2.5*3.6 其中数字和运算符之间有一个空格隔开。 编写python程序:读取data.txt文件中的每一行,并进行运算,将结果按行保存在当前目录中的resul.txt文件中。
好的,我可以帮你编写这个程序。以下是代码示例:
```python
with open('data.txt', 'r') as f:
lines = f.readlines()
results = []
for line in lines:
num1, op, num2 = line.split()
num1, num2 = float(num1), float(num2)
if op == '+':
result = num1 + num2
elif op == '-':
result = num1 - num2
elif op == '*':
result = num1 * num2
elif op == '/':
result = num1 / num2
else:
raise ValueError(f"Invalid operator: {op}")
results.append(str(result))
with open('result.txt', 'w') as f:
f.write('\n'.join(results))
```
这个程序会读取当前目录下的 `data.txt` 文件,并逐行进行数学运算,将结果保存在 `result.txt` 文件中。注意,在每行的计算结果保存时,要将结果转换为字符串格式。
阅读全文
相关推荐
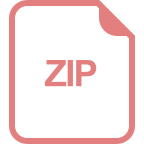











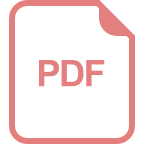
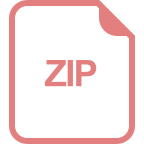