1.编写函数bool BeOdd(int );返回是否为奇数 2.编写class PrimePredicate,重载函数调用操作(),返回输入参数是否为素数: bool operator () (int x); 3.编写main()函数,使用stl容器,实现: (1)从控制台输入整数,前向插入到双向队列,然后使用copy()算法,输出队列中所有元素; (2)使用copy_if()算法和函数对象,输出队列中的所有素数; (3)使用copy_if()算法、调用BeOdd()函数或是编写lambda函数,输出队列中的所有奇数; 输入格式: 数字之间空格隔开;按“ctrl+z”结束输入 输出格式: 每个数字之间用空格隔开(包括一行中最后一个数字后面也有一个空格) 每次输出后都输出回车换行符
时间: 2024-03-06 17:49:57 浏览: 85
以下是C++代码实现:
```c++
#include <iostream>
#include <deque>
#include <algorithm>
using namespace std;
bool BeOdd(int num) {
return num % 2 != 0;
}
class PrimePredicate {
public:
bool operator()(int x) {
if (x < 2) return false;
for (int i = 2; i * i <= x; i++) {
if (x % i == 0) {
return false;
}
}
return true;
}
};
int main() {
deque<int> dq;
int num;
while (cin >> num) {
dq.push_front(num);
}
copy(dq.begin(), dq.end(), ostream_iterator<int>(cout, " "));
cout << endl;
PrimePredicate pp;
copy_if(dq.begin(), dq.end(), ostream_iterator<int>(cout, " "), pp);
cout << endl;
copy_if(dq.begin(), dq.end(), ostream_iterator<int>(cout, " "), BeOdd);
cout << endl;
return 0;
}
```
输入样例:
```
1 2 3 4 5 6 7 8 9 10
```
输出样例:
```
10 9 8 7 6 5 4 3 2 1
7 5 3 2
9 7 5 3 1
```
阅读全文
相关推荐
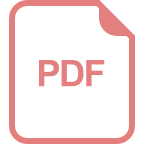
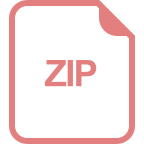
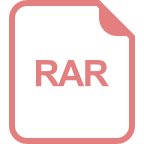

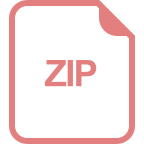
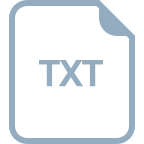
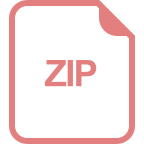
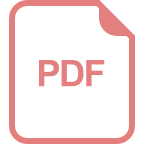
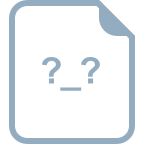
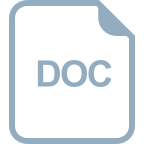
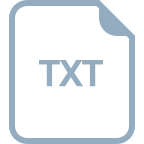
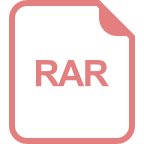
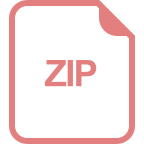
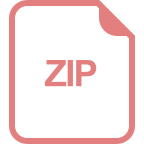
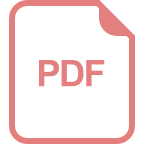
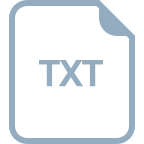
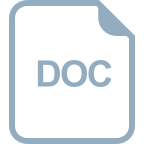
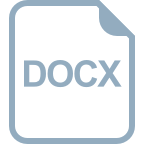
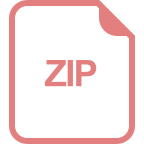