完成01-gravity.html 并注释关键代码
时间: 2023-06-21 17:12:50 浏览: 50
以下是01-gravity.html的代码及注释:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Gravity Simulation</title>
<!-- 引入样式文件 -->
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<!-- 创建画布 -->
<canvas id="canvas"></canvas>
<!-- 引入JavaScript文件 -->
<script src="main.js"></script>
</body>
</html>
```
```css
/* style.css */
/* 设置画布样式 */
canvas {
background-color: #f2f2f2;
width: 100%;
height: 100%;
display: block;
position: absolute;
top: 0;
left: 0;
}
```
```javascript
// main.js
// 获取画布和上下文
var canvas = document.getElementById('canvas');
var ctx = canvas.getContext('2d');
// 设置画布大小
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
// 创建星球类
class Planet {
constructor(x, y, dx, dy, radius, color) {
this.x = x; // x 坐标
this.y = y; // y 坐标
this.dx = dx; // x 方向速度
this.dy = dy; // y 方向速度
this.radius = radius; // 半径
this.color = color; // 颜色
}
// 绘制星球
draw() {
ctx.beginPath();
ctx.arc(this.x, this.y, this.radius, 0, Math.PI * 2, false);
ctx.fillStyle = this.color;
ctx.fill();
ctx.closePath();
}
// 更新星球位置
update() {
// 碰到边缘时反弹
if (this.x + this.radius > canvas.width || this.x - this.radius < 0) {
this.dx = -this.dx;
}
if (this.y + this.radius > canvas.height || this.y - this.radius < 0) {
this.dy = -this.dy;
}
// 更新位置
this.x += this.dx;
this.y += this.dy;
// 绘制星球
this.draw();
}
}
// 创建星球数组
var planets = [];
// 创建 20 个星球
for (var i = 0; i < 20; i++) {
var radius = Math.random() * 50 + 10; // 随机半径
var x = Math.random() * (canvas.width - radius * 2) + radius; // 随机 x 坐标
var y = Math.random() * (canvas.height - radius * 2) + radius; // 随机 y 坐标
var dx = (Math.random() - 0.5) * 10; // 随机 x 方向速度
var dy = (Math.random() - 0.5) * 10; // 随机 y 方向速度
var color = `rgb(${Math.random() * 255},${Math.random() * 255},${Math.random() * 255})`; // 随机颜色
planets.push(new Planet(x, y, dx, dy, radius, color)); // 添加到星球数组
}
// 动画循环
function animate() {
requestAnimationFrame(animate);
ctx.clearRect(0, 0, canvas.width, canvas.height); // 清空画布
// 更新每个星球的位置
for (var i = 0; i < planets.length; i++) {
planets[i].update();
}
}
// 开始动画
animate();
```
注释中涉及到的一些关键代码:
- `canvas.getContext('2d')`: 获取画布上下文,返回一个`CanvasRenderingContext2D`对象,可以用于绘制 2D 图形。
- `ctx.beginPath()`: 启动一条新路径或重置当前路径。
- `ctx.arc(x, y, radius, startAngle, endAngle, anticlockwise)`: 绘制一条以`(x, y)`为圆心、半径为`radius`的圆弧,起点和终点角度由`startAngle`和`endAngle`指定,如果`anticlockwise`为`true`则逆时针绘制,否则顺时针绘制。
- `ctx.fillStyle`: 设置绘制图形的填充颜色。
- `ctx.fill()`: 填充当前路径。
- `requestAnimationFrame(callback)`: 请求浏览器在下一次重绘前调用`callback`函数,通常用于实现动画效果。
- `ctx.clearRect(x, y, width, height)`: 清空矩形区域,左上角坐标为`(x, y)`,宽高分别为`width`和`height`。
相关推荐
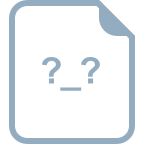















