package jdbc.score.mapper; import java.sql.ResultSet; import java.sql.SQLException; import org.springframework.jdbc.core.RowMapper; import jdbc.score.entities.Student; public class StudentMapper implements RowMapper<Student> { //自定义映射规则 public Student mapRow(ResultSet arg0, int arg1) throws SQLException { // TODO Auto-generated method stub } }
时间: 2024-03-04 17:53:22 浏览: 23
这是一段 Java 代码,是使用 Spring JDBC 操作数据库时的一个映射类。其中,`StudentMapper` 实现了 `RowMapper` 接口,用于将查询结果集中的一行记录转换为 `Student` 对象。在 `mapRow()` 方法中,可以自定义映射规则,将查询结果中的字段值与 `Student` 对象的属性进行映射。具体实现方式可以根据项目需求不同而不同。
相关问题
package jdbc.score.dao.impl; import java.util.List; import org.springframework.jdbc.core.JdbcTemplate; import jdbc.score.dao.StudentDao; import jdbc.score.entities.Student; import jdbc.score.mapper.StudentMapper; public class StudentDaoImpl implements StudentDao { JdbcTemplate jdbct; public JdbcTemplate getJdbct() { return jdbct; } public void setJdbct(JdbcTemplate jdbct) { this.jdbct = jdbct; } //根据班级查找学生信息(支持模糊查找) @Override ...........(代码2) }
好的,根据您提供的代码,代码2的部分应该是这样的:
```java
@Override
public List<Student> findByClass(String className) {
String sql = "SELECT * FROM student WHERE class_name LIKE ?";
Object[] args = new Object[]{"%" + className + "%"};
List<Student> students = jdbct.query(sql, args, new StudentMapper());
return students;
}
```
这个方法可以根据传入的班级名(模糊匹配)查询学生信息,并返回一个学生对象列表。具体实现中使用了JdbcTemplate的query方法,传入SQL语句和参数数组,并使用StudentMapper将结果映射到Student对象中。
Caused by: java.lang.ClassNotFoundException: org.springframework.data.elasticsearch.core.EntityMapper
Caused by: java.lang.ClassNotFoundException: org.springframework.data.elasticsearch.core.EntityMapper 是一个Java异常,表示在运行时找不到org.springframework.data.elasticsearch.core.EntityMapper类。
这个异常通常发生在使用Spring Data Elasticsearch时,由于缺少相关的依赖或配置问题导致无法找到EntityMapper类。
要解决这个异常,你可以尝试以下几个步骤:
1. 确保你的项目中包含了正确的Spring Data Elasticsearch依赖。你可以在项目的构建文件(如pom.xml或build.gradle)中添加相应的依赖。
2. 检查你的项目配置文件,确保已正确配置了Elasticsearch相关的连接信息和实体映射配置。你可以参考Spring Data Elasticsearch的官方文档来了解正确的配置方式。
3. 如果你使用的是Spring Boot,可以尝试重新启动应用程序,以确保所有的依赖都正确加载。
4. 如果以上步骤都没有解决问题,可能是由于版本不兼容或其他原因导致。你可以尝试更新相关的依赖版本,或者查找其他开发者在类似情况下的解决方案。
希望以上信息对你有帮助!如果还有其他问题,请继续提问。
相关推荐
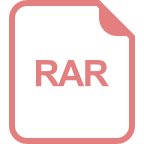












