如何获取到参数为HttpServletResponse response中的文件,并将文件保存到指定的位置,封装成可直接使用且不会报错的工具类
时间: 2023-07-16 08:15:52 浏览: 51
可以使用如下的工具类来实现:
```java
import javax.servlet.http.HttpServletResponse;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.net.URLEncoder;
public class FileDownloadUtil {
/**
* 下载文件
*
* @param response HttpServletResponse对象
* @param filePath 文件路径
* @param fileName 文件名
* @throws Exception
*/
public static void download(HttpServletResponse response, String filePath, String fileName) throws Exception {
// 设置响应类型为二进制流
response.setContentType("application/octet-stream;charset=UTF-8");
// 设置响应头信息
response.setHeader("Content-Disposition", "attachment;filename=" + URLEncoder.encode(fileName, "UTF-8"));
// 创建文件对象
File file = new File(filePath);
// 创建文件输入流
BufferedInputStream bis = new BufferedInputStream(new FileInputStream(file));
// 创建输出流
BufferedOutputStream bos = new BufferedOutputStream(response.getOutputStream());
// 缓冲区
byte[] buffer = new byte[1024];
int len = 0;
// 读取文件并输出到客户端
while ((len = bis.read(buffer)) != -1) {
bos.write(buffer, 0, len);
}
// 关闭输出流和输入流
bos.close();
bis.close();
}
/**
* 保存文件
*
* @param inputStream 输入流
* @param filePath 文件路径
* @param fileName 文件名
* @throws Exception
*/
public static void saveFile(InputStream inputStream, String filePath, String fileName) throws Exception {
// 创建文件对象
File file = new File(filePath);
if (!file.exists()) {
// 若文件目录不存在,则创建目录
file.mkdirs();
}
// 创建文件输出流
FileOutputStream fos = new FileOutputStream(filePath + File.separator + fileName);
// 创建缓冲区
byte[] buffer = new byte[1024];
int len = 0;
// 读取输入流并保存到文件
while ((len = inputStream.read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
// 关闭输入流和输出流
fos.close();
inputStream.close();
}
}
```
其中,`download` 方法实现了从服务器下载文件的功能,`saveFile` 方法实现了将文件保存到指定位置的功能。这两个方法都是静态方法,可以直接调用。需要注意的是,在调用 `download` 方法前,需要设置好文件的路径和文件名;在调用 `saveFile` 方法前,需要将文件的输入流、路径和文件名传入。此外,下载时还需要将 HttpServletResponse 对象作为参数传入。
相关推荐
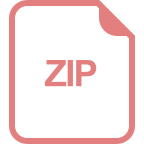
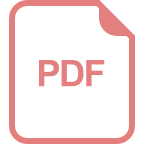
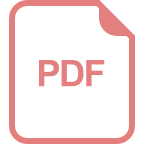














