用Python写一个程序,输入年月日,输出该日期是这年的第几天
时间: 2023-05-13 09:05:43 浏览: 80
以下是Python代码:
```python
def is_leap_year(year):
return year % 4 == 0 and (year % 100 != 0 or year % 400 == 0)
def day_of_year(year, month, day):
days_of_month = [0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
if is_leap_year(year):
days_of_month[2] = 29
total_days = sum(days_of_month[:month]) + day
return total_days
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
day = int(input("请输入日期:"))
print("该日期是这年的第%d天" % day_of_year(year, month, day))
```
输入年月日后,程序会输出该日期是这年的第几天。
相关问题
用Python写一个程序,输入年月日,输某年某月某日是这年的第几天
以下是Python代码:
```python
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
day = int(input("请输入日期:"))
days_in_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
if year % 4 == 0 and (year % 100 != 0 or year % 400 == 0):
days_in_month[1] = 29
day_count = sum(days_in_month[:month-1]) + day
print("这是{}年的第{}天。".format(year, day_count))
```
输入年月日后,程序会计算出这是这一年的第几天,并输出结果。
从键盘输入年月日,输出这是这一年的第多少天,用 python编程输出
以下是Python代码:
```python
# 获取日期
date_str = input("请输入日期(格式:YYYY-MM-DD): ")
# 将日期字符串转换为日期对象
date_obj = datetime.datetime.strptime(date_str, "%Y-%m-%d")
# 获取该日期是该年的第几天
day_of_year = date_obj.timetuple().tm_yday
# 输出结果
print("{}是{}年的第{}天".format(date_str, date_obj.year, day_of_year))
```
说明:
1. 程序通过`input`函数获取输入的日期字符串
2. 使用`datetime`模块中的`strptime`函数将日期字符串转换为日期对象
3. 使用`timetuple()`函数将日期对象转换为一元组,其中包含了年、月、日等信息。使用其中的`tm_yday`属性获取该日期是该年的第几天。
4. 使用字符串格式化输出结果。
相关推荐
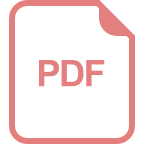












