java获取明天下午2点的时间戳
时间: 2024-01-29 18:02:48 浏览: 79
可以使用以下代码获取明天下午2点的时间戳:
```
Calendar calendar = Calendar.getInstance();
calendar.add(Calendar.DATE, 1); // 获取明天的日期
calendar.set(Calendar.HOUR_OF_DAY, 14); // 设置小时为下午2点
calendar.set(Calendar.MINUTE, 0); // 设置分钟为0
calendar.set(Calendar.SECOND, 0); // 设置秒数为0
calendar.set(Calendar.MILLISECOND, 0); // 设置毫秒数为0
long timestamp = calendar.getTimeInMillis() / 1000; // 将毫秒数转换为秒数
System.out.println("明天下午2点的时间戳为:" + timestamp);
```
注意,这里获取的时间戳是以秒为单位的。如果需要以毫秒为单位,可以直接使用`calendar.getTimeInMillis()`来获取时间戳。
相关问题
java 获取随机时间戳不是固定时间戳
如果你想获取一个随机的时间戳,可以使用Java的Random类来生成一个随机的时间戳,代码如下:
```java
import java.util.Random;
Random random = new Random();
long timestamp = System.currentTimeMillis() - random.nextInt(1000000);
System.out.println(timestamp);
```
其中,System.currentTimeMillis()方法返回的是当前时间距离1970年1月1日00:00:00经过的毫秒数,而Random.nextInt(n)方法返回一个0到n-1的随机整数,这里我们使用这个方法来生成一个0到999999之间的随机整数,然后将其减去当前时间戳,即可得到一个随机的时间戳。
java 获取30天前的时间戳
### 回答1:
可以使用Java的`java.time`包中的`LocalDateTime`和`Instant`类来获取30天前的时间戳。具体代码如下:
```java
import java.time.LocalDateTime;
import java.time.Instant;
import java.time.temporal.ChronoUnit;
public class Example {
public static void main(String[] args) {
// 获取当前时间
LocalDateTime currentDateTime = LocalDateTime.now();
// 获取30天前的时间
LocalDateTime beforeDateTime = currentDateTime.minus(30, ChronoUnit.DAYS);
// 转换为时间戳
Instant beforeInstant = beforeDateTime.toInstant(ZoneOffset.UTC);
long beforeTimestamp = beforeInstant.toEpochMilli();
System.out.println("30天前的时间戳:" + beforeTimestamp);
}
}
```
上述代码中,我们首先获取当前时间`currentDateTime`,然后使用`minus()`方法获取30天前的时间`beforeDateTime`,接着使用`toInstant()`方法将其转换为`Instant`类型的时间戳,最后使用`toEpochMilli()`方法获取毫秒级时间戳。
### 回答2:
在Java中获取30天前的时间戳可以通过以下步骤实现。
首先,可以使用`java.time`包中的`LocalDateTime`类来表示当前时间。我们可以使用`LocalDateTime.now()`方法来获取当前的日期和时间。
接下来,我们可以使用`minusDays()`方法来减去30天。具体步骤如下:
```java
import java.time.LocalDateTime;
public class Main {
public static void main(String[] args) {
LocalDateTime currentDateTime = LocalDateTime.now(); // 获取当前时间
LocalDateTime thirtyDaysAgo = currentDateTime.minusDays(30); // 减去30天
long timestamp = thirtyDaysAgo.toEpochSecond(java.time.OffsetDateTime.now().getOffset()); // 将时间转换为时间戳
System.out.println(timestamp); // 输出时间戳
}
}
```
在上述代码中,我们首先获取当前时间并存储在`currentDateTime`变量中。然后使用`minusDays(30)`方法来减去30天,并将结果存储在`thirtyDaysAgo`变量中。最后,我们使用`toEpochSecond()`方法将`thirtyDaysAgo`转换为时间戳,并存储在`timestamp`变量中。
最后,我们可以使用`System.out.println()`来打印出获取到的时间戳。
运行上述代码,将会输出30天前的时间戳。
### 回答3:
Java中获取30天前的时间戳可以使用以下代码:
```java
import java.time.LocalDate;
import java.time.ZoneOffset;
public class Main {
public static void main(String[] args) {
// 获取当前日期
LocalDate currentDate = LocalDate.now();
// 获取30天前的日期
LocalDate thirtyDaysBefore = currentDate.minusDays(30);
// 将日期转换为时间戳(以秒为单位)
long timestamp = thirtyDaysBefore.atStartOfDay().toEpochSecond(ZoneOffset.UTC);
System.out.println(timestamp);
}
}
```
首先,我们使用`LocalDate.now()`获取当前的日期。然后,使用`minusDays(30)`方法从当前日期减去30天,得到30天前的日期。接下来,我们将这个日期转换为时间戳,这里使用的是`atStartOfDay().toEpochSecound(ZoneOffset.UTC)`方法,将日期转换为以UTC时区为基准的秒数的时间戳。最后,我们将时间戳打印出来。
以上代码的输出将是30天前的时间戳。
阅读全文
相关推荐
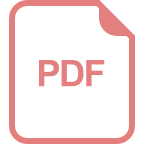
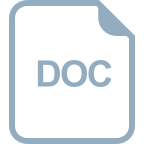











