vue3 滚动到某一位置显示某个元素并且添加动画,再次滚动时隐藏上个元素,显示下个元素
时间: 2024-02-21 09:01:36 浏览: 100
可以使用Vue3的`Intersection Observer` API来实现这个效果。首先,在需要显示的元素上添加`ref`引用,以及一个`v-if`指令来控制它的显示和隐藏:
```html
<div v-if="showElement" ref="myElement">要显示的元素</div>
```
然后,在Vue3组件的`setup()`函数中,使用`ref`来定义一个`Intersection Observer`对象,用来观察指定元素是否进入或离开了视口。在回调函数中,可以根据元素的可见性来设置`showElement`的值,从而控制元素的显示和隐藏:
```javascript
import { ref, onMounted } from 'vue'
export default {
setup() {
const observer = ref(null)
const showElement = ref(false)
const options = {
rootMargin: '0px',
threshold: 0.5
}
const callback = (entries, observer) => {
const [entry] = entries
if (entry.isIntersecting) {
showElement.value = true
observer.unobserve(entry.target)
}
}
onMounted(() => {
observer.value = new IntersectionObserver(callback, options)
observer.value.observe(myElement.value)
})
return { showElement, myElement }
}
}
```
最后,为了实现动画效果,可以在元素上添加一个`transition`属性,以及一个`watch`监听`showElement`的变化,来触发动画:
```html
<template>
<div>
<div v-if="showElement" ref="myElement" class="animate__animated animate__fadeInDown">要显示的元素</div>
</div>
</template>
<script>
import { ref, onMounted, watch } from 'vue'
export default {
setup() {
const observer = ref(null)
const showElement = ref(false)
const options = {
rootMargin: '0px',
threshold: 0.5
}
const callback = (entries, observer) => {
const [entry] = entries
if (entry.isIntersecting) {
showElement.value = true
observer.unobserve(entry.target)
}
}
const myElement = ref(null)
onMounted(() => {
observer.value = new IntersectionObserver(callback, options)
observer.value.observe(myElement.value)
})
watch(showElement, (newValue, oldValue) => {
if (oldValue === true && newValue === false) {
myElement.value.classList.remove('animate__fadeInDown')
myElement.value.classList.add('animate__fadeOutUp')
}
else if (oldValue === false && newValue === true) {
myElement.value.classList.remove('animate__fadeOutUp')
myElement.value.classList.add('animate__fadeInDown')
}
})
return { showElement, myElement }
}
}
</script>
<style>
.animate__animated {
animation-duration: 1s;
animation-fill-mode: both;
}
.animate__fadeInDown {
animation-name: fadeInDown;
}
.animate__fadeOutUp {
animation-name: fadeOutUp;
}
@keyframes fadeInDown {
from {
opacity: 0;
transform: translate3d(0, -100%, 0);
}
to {
opacity: 1;
transform: none;
}
}
@keyframes fadeOutUp {
from {
opacity: 1;
transform: none;
}
to {
opacity: 0;
transform: translate3d(0, -100%, 0);
}
}
</style>
```
这样,当元素进入视口时,它就会以`fadeInDown`动画显示出来;当元素离开视口时,它就会以`fadeOutUp`动画隐藏起来。
阅读全文
相关推荐
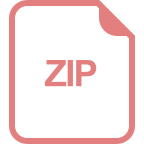
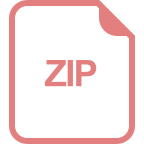
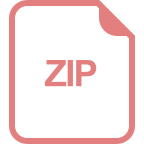
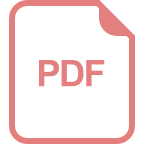
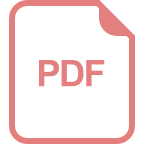
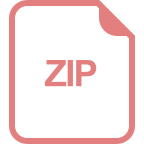
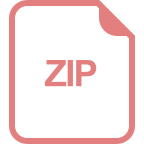
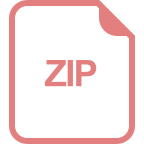
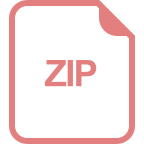
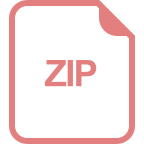
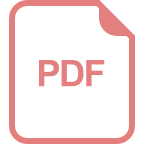
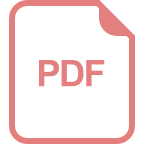
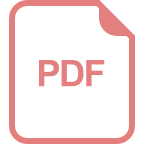
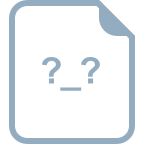
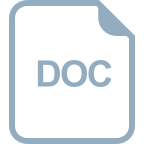
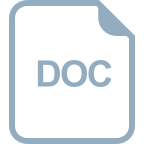
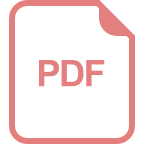