vue自定义生成可编辑的票据、单据模板的插件
时间: 2024-01-08 18:04:22 浏览: 36
如果需要在多个项目中使用自定义生成可编辑的票据、单据模板的功能,可以将它封装成一个Vue.js插件,方便在不同的应用程序中复用。下面是一个简单的插件示例,帮助你了解如何实现自定义生成可编辑的票据、单据模板的插件。
1. 创建一个插件文件,例如my-invoice.js,导出一个对象,包含插件的名称、版本号和安装函数。
```javascript
export default {
name: 'my-invoice',
version: '1.0.0',
install: function(Vue, options) {
Vue.component('my-invoice', {
props: ['data'],
data: function() {
return {
invoice: this.data
};
},
methods: {
addItem: function() {
this.invoice.items.push({ name: '', price: '', quantity: '', amount: '' });
},
removeItem: function(index) {
this.invoice.items.splice(index, 1);
}
},
template: `
<div>
<h2>{{ invoice.title }}</h2>
<p>单号:{{ invoice.number }}</p>
<p>日期:{{ invoice.date }}</p>
<table>
<thead>
<tr>
<th>名称</th>
<th>单价</th>
<th>数量</th>
<th>金额</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in invoice.items" :key="index">
<td><input type="text" v-model="item.name"></td>
<td><input type="text" v-model="item.price"></td>
<td><input type="text" v-model="item.quantity"></td>
<td><input type="text" v-model="item.amount"></td>
<td><button @click="removeItem(index)">删除</button></td>
</tr>
</tbody>
<tfoot>
<tr>
<td colspan="3">合计</td>
<td><input type="text" v-model="invoice.total"></td>
<td><button @click="addItem">添加行</button></td>
</tr>
</tfoot>
</table>
</div>
`
});
}
};
```
2. 在需要使用插件的项目中,导入插件并安装。
```javascript
import Vue from 'vue';
import MyInvoice from './my-invoice';
Vue.use(MyInvoice);
```
3. 在Vue组件中使用插件,并传递数据。
```html
<template>
<div>
<my-invoice :data="invoice"></my-invoice>
</div>
</template>
<script>
export default {
data: function() {
return {
invoice: {
title: '发票',
number: '',
date: '',
items: [
{ name: '', price: '', quantity: '', amount: '' }
],
total: ''
}
};
}
};
</script>
```
总之,将自定义生成可编辑的票据、单据模板封装成Vue.js插件,可以方便地在多个项目中复用,并提高开发效率和代码可维护性。插件的实现方式可以根据具体的需求和场景进行定制,可以使用组件、指令、过滤器和混入等技术实现。
相关推荐
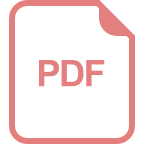














