java excel自动合并行单元格
时间: 2023-09-18 14:08:40 浏览: 56
以下是使用Apache POI库在Java中自动合并Excel行单元格的示例代码:
```
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.CellStyle;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.WorkbookFactory;
public class ExcelMergeRows {
public static void main(String[] args) {
try {
// 读取Excel文件
FileInputStream fis = new FileInputStream(new File("input.xlsx"));
Workbook workbook = WorkbookFactory.create(fis);
Sheet sheet = workbook.getSheetAt(0);
// 定义要合并的列
int mergeColumn = 0; // 第一列
// 遍历所有行
int rowCount = sheet.getLastRowNum();
for (int i = 0; i <= rowCount; i++) {
Row row = sheet.getRow(i);
if (row == null) {
continue;
}
// 获取当前单元格和上一个单元格的值
Cell currentCell = row.getCell(mergeColumn);
Cell prevCell = null;
if (i > 0) {
prevCell = sheet.getRow(i - 1).getCell(mergeColumn);
}
// 如果当前单元格和上一个单元格的值相同,则合并单元格
if (currentCell != null && prevCell != null && currentCell.toString().equals(prevCell.toString())) {
CellStyle style = currentCell.getCellStyle();
sheet.getRow(i - 1).getCell(mergeColumn).setCellStyle(style);
sheet.addMergedRegion(new org.apache.poi.ss.util.CellRangeAddress(i - 1, i, mergeColumn, mergeColumn));
}
}
// 保存Excel文件
FileOutputStream fos = new FileOutputStream(new File("output.xlsx"));
workbook.write(fos);
fis.close();
fos.close();
workbook.close();
System.out.println("Excel merged successfully!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这个示例代码假设要合并Excel文件的第一列。您可以通过更改变量`mergeColumn`来指定要合并的列。运行此代码后,将在当前目录下创建一个名为`output.xlsx`的新文件,其中包含已合并的单元格。
相关推荐
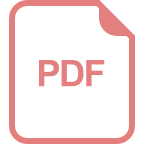














