帮我用vue 和css写一个类似微信通讯录的界面
时间: 2024-04-05 21:32:11 浏览: 168
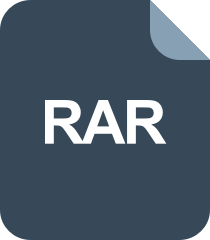
仿微信界面,含通讯录功能
好的,我可以给你提供一个简单的思路来实现类似微信通讯录的界面,使用 Vue 和 CSS 来实现。
首先,你需要创建一个 Vue 组件来表示通讯录界面。在这个组件中,你可以使用 Vue 的 data 属性来存储通讯录中的联系人信息,例如姓名、头像、电话号码等。
然后,你可以使用 CSS 来布局和样式化你的通讯录界面。你可以使用 flexbox 布局来创建一个列布局,每列表示一个字母,用于分组显示联系人。你可以使用 CSS 样式来设计每个联系人的卡片,包括头像、姓名和电话号码等信息。
为了实现搜索功能,你可以在组件中添加一个搜索框,使用 Vue 的 computed 属性来过滤联系人信息,只显示与搜索关键字匹配的联系人。
最后,你可以添加一些交互效果,例如当用户点击一个联系人时,该联系人的详细信息会在侧边栏中显示出来。
以下是一个简单的代码示例,用于实现类似微信通讯录的界面:
```
<template>
<div class="contacts">
<div class="search">
<input type="text" v-model="searchKeyword" placeholder="Search...">
</div>
<div class="columns">
<div v-for="letter in letters" :key="letter" class="column">
<div class="letter">{{ letter }}</div>
<div v-for="contact in filteredContacts" :key="contact.id" class="contact">
<img :src="contact.avatar" alt="Avatar">
<div class="info">
<div class="name">{{ contact.name }}</div>
<div class="phone">{{ contact.phone }}</div>
</div>
</div>
</div>
</div>
<div v-if="selectedContact" class="sidebar">
<img :src="selectedContact.avatar" alt="Avatar">
<div class="info">
<div class="name">{{ selectedContact.name }}</div>
<div class="phone">{{ selectedContact.phone }}</div>
</div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
contacts: [
{ id: 1, name: 'Alice', phone: '123-456-7890', avatar: 'https://randomuser.me/api/portraits/women/1.jpg' },
{ id: 2, name: 'Bob', phone: '456-789-0123', avatar: 'https://randomuser.me/api/portraits/men/2.jpg' },
{ id: 3, name: 'Charlie', phone: '789-012-3456', avatar: 'https://randomuser.me/api/portraits/men/3.jpg' },
// ...
],
selectedContact: null,
searchKeyword: ''
}
},
computed: {
letters() {
const letters = new Set()
this.contacts.forEach(contact => {
letters.add(contact.name.charAt(0).toUpperCase())
})
return Array.from(letters).sort()
},
filteredContacts() {
if (!this.searchKeyword) {
return this.contacts
}
const keyword = this.searchKeyword.toLowerCase()
return this.contacts.filter(contact => {
return contact.name.toLowerCase().indexOf(keyword) !== -1
})
}
},
methods: {
selectContact(contact) {
this.selectedContact = contact
}
}
}
</script>
<style>
.contacts {
display: flex;
flex-direction: row;
justify-content: center;
align-items: center;
height: 100vh;
font-size: 16px;
}
.search {
margin-bottom: 16px;
}
.columns {
display: flex;
flex-direction: row;
justify-content: center;
align-items: flex-start;
flex-wrap: wrap;
width: 80%;
}
.column {
display: flex;
flex-direction: column;
margin-right: 16px;
}
.letter {
font-weight: bold;
margin-bottom: 8px;
}
.contact {
display: flex;
flex-direction: row;
align-items: center;
margin-bottom: 8px;
cursor: pointer;
}
.contact:hover {
background-color: #eee;
}
.contact img {
width: 48px;
height: 48px;
border-radius: 50%;
margin-right: 16px;
}
.info {
display: flex;
flex-direction: column;
}
.name {
font-weight: bold;
}
.phone {
color: #888;
}
.sidebar {
position: fixed;
top: 0;
right: 0;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
width: 20%;
height: 100%;
background-color: #f7f7f7;
box-shadow: 0 0 16px rgba(0, 0, 0, 0.1);
}
.sidebar img {
width: 128px;
height: 128px;
border-radius: 50%;
margin-bottom: 16px;
}
.sidebar .info {
display: flex;
flex-direction: column;
align-items: center;
}
.sidebar .name {
font-size: 24px;
font-weight: bold;
margin-bottom: 8px;
}
.sidebar .phone {
color: #888;
}
</style>
```
阅读全文
相关推荐
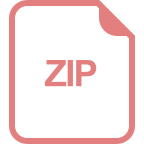





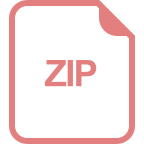
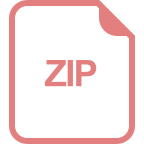
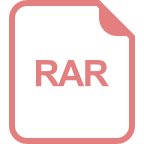
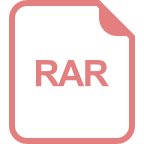
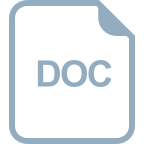
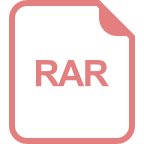
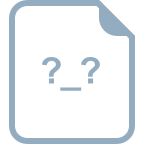
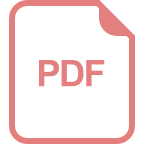
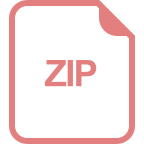
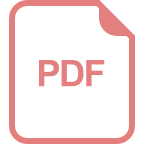
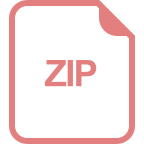