kmeans rssi matlab
时间: 2023-10-16 07:22:22 浏览: 43
K-means 是一种无监督学习算法,可以将一组数据分成多个簇。RSSI(Received Signal Strength Indicator)是接收信号强度指示,用于衡量接收器接收到的无线信号的强度。在 MATLAB 中,可以使用 kmeans 函数实现对 RSSI 数据的聚类。
下面是一个简单的 MATLAB 代码示例,用于对一组 RSSI 数据进行聚类:
```matlab
% 导入 RSSI 数据,数据存储在 rssi.mat 文件中
load rssi.mat
% 设置聚类数目
k = 3;
% 运行 k-means 算法
[idx, C] = kmeans(rssi, k);
% 绘制聚类结果
scatter(rssi(:,1), rssi(:,2), 10, idx, 'filled')
hold on
plot(C(:,1), C(:,2), 'kx', 'MarkerSize', 15, 'LineWidth', 3)
hold off
```
在上述代码中,我们首先导入 RSSI 数据,然后设置聚类数目为 3。接着,我们使用 kmeans 函数对数据进行聚类,得到每个数据点所属的簇编号 idx 和聚类中心 C。最后,我们使用 scatter 函数将数据点按簇编号进行绘制,并用 plot 函数绘制聚类中心。
需要注意的是,上述代码仅为示例,实际应用中需要根据具体数据进行调整。
相关问题
kmeans函数matlab
在 MATLAB 中,可以使用 `kmeans` 函数进行 K-means 聚类。该函数的语法如下:
```matlab
[idx, C] = kmeans(X, k)
```
其中,`X` 为一个大小为 `m-by-n` 的矩阵,表示 m 个 n 维样本的特征向量;`k` 为聚类的个数;`idx` 为大小为 `m-by-1` 的一维向量,表示每个样本所属的聚类的索引;`C` 为大小为 `k-by-n` 的矩阵,表示聚类的中心点。
另外,`kmeans` 函数还有其他的输入参数和输出参数,可以根据实际需要进行使用。例如,可以通过指定 `'Distance'` 参数来选择不同的距离度量方式,例如欧几里得距离或曼哈顿距离等。
下面是一个使用 `kmeans` 函数进行聚类的示例:
```matlab
% 生成随机数据
X = [randn(100, 2)*0.75+ones(100, 2);
randn(100, 2)*0.5-ones(100, 2)];
% 使用 kmeans 函数进行聚类
[idx, C] = kmeans(X, 2);
% 可视化聚类结果
figure;
plot(X(idx==1,1),X(idx==1,2),'r.','MarkerSize',12)
hold on
plot(X(idx==2,1),X(idx==2,2),'b.','MarkerSize',12)
plot(C(:,1),C(:,2),'kx', 'MarkerSize',15,'LineWidth',3)
legend('Cluster 1','Cluster 2','Centroids','Location','NW')
title 'K-means Clustering'
hold off
```
该示例代码生成了一个大小为 `200-by-2` 的随机数据矩阵 `X`,然后使用 `kmeans` 函数将数据聚为 2 类,并将聚类结果可视化。
kmeans代码matlab
Sure, here is an example of k-means clustering algorithm implemented in MATLAB:
```matlab
% Generate some random data
data = rand(100, 2);
% Set the number of clusters
k = 3;
% Initialize the centroids randomly
centroids = data(randperm(size(data, 1), k), :);
% Run the k-means algorithm
for i = 1:10
% Assign each data point to the nearest centroid
[~, labels] = pdist2(centroids, data, 'euclidean', 'Smallest', 1);
% Update the centroids
for j = 1:k
centroids(j, :) = mean(data(labels == j, :), 1);
end
end
% Plot the results
scatter(data(:, 1), data(:, 2), [], labels);
hold on;
scatter(centroids(:, 1), centroids(:, 2), 100, 'k', 'filled');
```
This code generates some random data and applies the k-means algorithm to cluster it into `k` clusters. The centroids are initialized randomly and updated iteratively until convergence. Finally, the results are plotted with different colors for each cluster and black circles for the centroids.
相关推荐
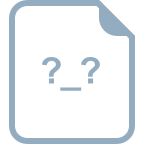
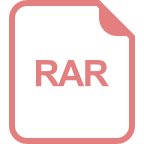












