用python制作一个gui界面
时间: 2023-03-25 21:03:51 浏览: 133
可以使用Python的Tkinter模块来制作GUI界面。首先需要导入Tkinter模块,然后创建一个窗口对象,添加各种组件,如按钮、标签、文本框等,最后使用mainloop()方法来显示窗口并等待用户操作。具体实现可以参考Tkinter的官方文档或者相关教程。
相关问题
用python制作一个有GUI界面的文本转语音工具
好的,这里是一个使用Python和Tkinter GUI框架的简单文本转语音工具的示例代码:
```python
import tkinter as tk
from gtts import gTTS
from playsound import playsound
def convert_text_to_speech():
text = text_input.get("1.0", "end-1c")
speech = gTTS(text)
speech.save("output.mp3")
playsound("output.mp3")
root = tk.Tk()
root.title("文本转语音工具")
text_label = tk.Label(root, text="请输入要转换的文本:")
text_label.pack()
text_input = tk.Text(root)
text_input.pack()
convert_button = tk.Button(root, text="转换", command=convert_text_to_speech)
convert_button.pack()
root.mainloop()
```
该程序使用了gTTS库来将文本转换为音频文件,并使用playsound库播放音频文件。在GUI界面中,用户可以输入要转换的文本,然后点击“转换”按钮即可将文本转换为音频并播放。
请注意,该程序只是一个简单的示例,您可以根据自己的需求进行修改和扩展。
用Python制作一个登录界面
可以使用Python的GUI库Tkinter来制作登录界面。
以下是一个简单的登录界面示例代码:
```python
from tkinter import *
from tkinter import messagebox
def login():
username = username_entry.get()
password = password_entry.get()
if username == "admin" and password == "123456":
messagebox.showinfo("Login", "Login Successful!")
else:
messagebox.showerror("Login", "Invalid Username or Password")
root = Tk()
root.title("Login")
user_label = Label(root, text="Username:")
user_label.grid(row=0, column=0)
username_entry = Entry(root)
username_entry.grid(row=0, column=1)
password_label = Label(root, text="Password:")
password_label.grid(row=1, column=0)
password_entry = Entry(root, show="*")
password_entry.grid(row=1, column=1)
login_button = Button(root, text="Login", command=login)
login_button.grid(row=2, column=1)
root.mainloop()
```
这个代码中,我们创建了一个名为“Login”的窗口,并在窗口中添加了两个标签、两个文本框和一个登录按钮。当用户点击登录按钮时,我们获取文本框中的用户名和密码,并使用简单的if-else语句进行验证。如果用户名和密码都正确,我们将弹出一个消息框,显示“登录成功”;否则,我们将弹出一个错误消息框,显示“用户名或密码无效”。
你可以根据自己的需要进行修改和扩展。
相关推荐
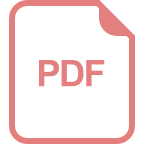
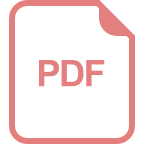












