存储器的分配与回收算法实现
时间: 2023-06-08 07:04:40 浏览: 189
存储器的分配与回收算法实现是计算机操作系统中的一个重要问题。常见的存储器分配算法有首次适应(First Fit)、最佳适应(Best Fit)、最差适应(Worst Fit)等。而回收算法则有标记清除(Mark and Sweep)、引用计数(Reference Counting)等。这些算法的实现细节会因不同的操作系统而异。具体的实现及调优方法可以参考相关文献或资料。
相关问题
存储器的分配与回收算法实现用java代码实现,源代码
以下是一些常见的内存分配和回收算法的Java代码实现:
1. 首次适应算法(First Fit)
```
public class FirstFitAllocation {
private int memorySize;
private int[] memory;
private boolean[] isAllocated;
public FirstFitAllocation(int memorySize) {
this.memorySize = memorySize;
memory = new int[memorySize];
isAllocated = new boolean[memorySize];
}
public int allocate(int size) {
for (int i = 0; i < memorySize; i++) {
if (!isAllocated[i] && i + size <= memorySize) {
boolean canAllocate = true;
for (int j = 0; j < size; j++) {
if (isAllocated[i + j]) {
canAllocate = false;
break;
}
}
if (canAllocate) {
for (int j = 0; j < size; j++) {
memory[i + j] = i;
isAllocated[i + j] = true;
}
return i;
}
}
}
return -1;
}
public void deallocate(int start, int size) {
for (int i = start; i < start + size; i++) {
memory[i] = 0;
isAllocated[i] = false;
}
}
}
```
2. 循环首次适应算法(Next Fit)
```
public class NextFitAllocation {
private int memorySize;
private int[] memory;
private boolean[] isAllocated;
private int lastAllocatedIndex;
public NextFitAllocation(int memorySize) {
this.memorySize = memorySize;
memory = new int[memorySize];
isAllocated = new boolean[memorySize];
lastAllocatedIndex = 0;
}
public int allocate(int size) {
for (int i = lastAllocatedIndex; i < memorySize; i++) {
if (!isAllocated[i] && i + size <= memorySize) {
boolean canAllocate = true;
for (int j = 0; j < size; j++) {
if (isAllocated[i + j]) {
canAllocate = false;
break;
}
}
if (canAllocate) {
for (int j = 0; j < size; j++) {
memory[i + j] = i;
isAllocated[i + j] = true;
}
lastAllocatedIndex = i;
return i;
}
}
}
for (int i = 0; i < lastAllocatedIndex; i++) {
if (!isAllocated[i] && i + size <= memorySize) {
boolean canAllocate = true;
for (int j = 0; j < size; j++) {
if (isAllocated[i + j]) {
canAllocate = false;
break;
}
}
if (canAllocate) {
for (int j = 0; j < size; j++) {
memory[i + j] = i;
isAllocated[i + j] = true;
}
lastAllocatedIndex = i;
return i;
}
}
}
return -1;
}
public void deallocate(int start, int size) {
for (int i = start; i < start + size; i++) {
memory[i] = 0;
isAllocated[i] = false;
}
}
}
```
3. 最佳适应算法(Best Fit)
```
public class BestFitAllocation {
private int memorySize;
private int[] memory;
private boolean[] isAllocated;
public BestFitAllocation(int memorySize) {
this.memorySize = memorySize;
memory = new int[memorySize];
isAllocated = new boolean[memorySize];
}
public int allocate(int size) {
int bestFitStart = -1;
int bestFitSize = memorySize + 1;
for (int i = 0; i < memorySize; i++) {
if (!isAllocated[i] && i + size <= memorySize) {
boolean canAllocate = true;
int currentBlockSize = 0;
for (int j = 0; j < size; j++) {
if (isAllocated[i + j]) {
canAllocate = false;
break;
}
currentBlockSize++;
}
if (canAllocate && currentBlockSize < bestFitSize) {
bestFitStart = i;
bestFitSize = currentBlockSize;
}
}
}
if (bestFitStart >= 0) {
for (int j = 0; j < size; j++) {
memory[bestFitStart + j] = bestFitStart;
isAllocated[bestFitStart + j] = true;
}
}
return bestFitStart;
}
public void deallocate(int start, int size) {
for (int i = start; i < start + size; i++) {
memory[i] = 0;
isAllocated[i] = false;
}
}
}
```
4. 最坏适应算法(Worst Fit)
```
public class WorstFitAllocation {
private int memorySize;
private int[] memory;
private boolean[] isAllocated;
public WorstFitAllocation(int memorySize) {
this.memorySize = memorySize;
memory = new int[memorySize];
isAllocated = new boolean[memorySize];
}
public int allocate(int size) {
int worstFitStart = -1;
int worstFitSize = 0;
for (int i = 0; i < memorySize; i++) {
if (!isAllocated[i] && i + size <= memorySize) {
boolean canAllocate = true;
int currentBlockSize = 0;
for (int j = 0; j < size; j++) {
if (isAllocated[i + j]) {
canAllocate = false;
break;
}
currentBlockSize++;
}
if (canAllocate && currentBlockSize > worstFitSize) {
worstFitStart = i;
worstFitSize = currentBlockSize;
}
}
}
if (worstFitStart >= 0) {
for (int j = 0; j < size; j++) {
memory[worstFitStart + j] = worstFitStart;
isAllocated[worstFitStart + j] = true;
}
}
return worstFitStart;
}
public void deallocate(int start, int size) {
for (int i = start; i < start + size; i++) {
memory[i] = 0;
isAllocated[i] = false;
}
}
}
```
阅读全文
相关推荐

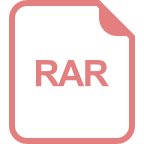










