怎么用c创建一个pdf文档
时间: 2024-03-20 22:44:50 浏览: 130
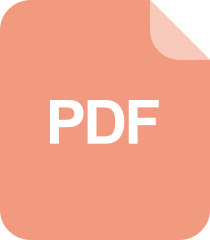
c语言创建文件的方法.pdf

要用C语言创建PDF文档,你可以使用一个名为libHaru的开源库。以下是一个简单的例子,演示如何使用libHaru创建一个包含一些文本和图形的PDF文档:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <math.h>
#include "hpdf.h"
void error_handler(HPDF_STATUS error_no, HPDF_STATUS detail_no, void *user_data) {
printf("Error: %04X, detail_no=%u\n", (unsigned int) error_no, (unsigned int) detail_no);
exit(-1);
}
int main(int argc, char **argv) {
HPDF_Doc pdf;
HPDF_Page page;
HPDF_Font font;
HPDF_REAL tw;
HPDF_REAL height;
HPDF_REAL width;
HPDF_REAL x;
HPDF_REAL y;
HPDF_STATUS status;
const char *font_name = "Helvetica-Bold";
const char *text = "Hello, world!";
const char *file_name = "example.pdf";
pdf = HPDF_New(error_handler, NULL);
if (!pdf) {
printf("Error: cannot create PDF object.\n");
return -1;
}
status = HPDF_SetCompressionMode(pdf, HPDF_COMP_ALL);
if (status != HPDF_OK) {
printf("Error: cannot set compression mode.\n");
HPDF_Free(pdf);
return -1;
}
status = HPDF_SetEncryptionMode(pdf, HPDF_ENCRYPT_R3, 16);
if (status != HPDF_OK) {
printf("Error: cannot set encryption mode.\n");
HPDF_Free(pdf);
return -1;
}
status = HPDF_SetPassword(pdf, "password", "owner_password");
if (status != HPDF_OK) {
printf("Error: cannot set password.\n");
HPDF_Free(pdf);
return -1;
}
font = HPDF_GetFont(pdf, font_name, NULL);
if (!font) {
printf("Error: cannot get font.\n");
HPDF_Free(pdf);
return -1;
}
height = HPDF_Page_GetHeight(page);
width = HPDF_Page_GetWidth(page);
page = HPDF_AddPage(pdf);
HPDF_Page_SetSize(page, HPDF_PAGE_SIZE_LETTER, HPDF_PAGE_PORTRAIT);
HPDF_Page_SetTextLeading(page, 20);
HPDF_Page_SetGrayFill(page, 0.5);
HPDF_Page_SetFontAndSize(page, font, 24);
tw = HPDF_Page_TextWidth(page, text);
x = (width - tw) / 2;
y = height - 50;
HPDF_Page_BeginText(page);
HPDF_Page_MoveTextPos(page, x, y);
HPDF_Page_ShowText(page, text);
HPDF_Page_EndText(page);
HPDF_Page_SetLineWidth(page, 1.0);
HPDF_Page_SetGrayStroke(page, 0.5);
HPDF_Page_Rectangle(page, 50, 50, width - 100, height - 100);
HPDF_Page_Stroke(page);
status = HPDF_SaveToFile(pdf, file_name);
if (status != HPDF_OK) {
printf("Error: cannot save PDF file.\n");
HPDF_Free(pdf);
return -1;
}
HPDF_Free(pdf);
return 0;
}
```
这段代码会创建一个包含一些文本和矩形的PDF文档,其中文本是居中对齐的,而矩形则在页面的中心。
阅读全文
相关推荐
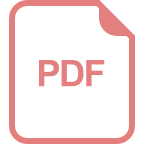
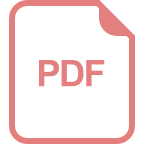
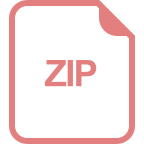
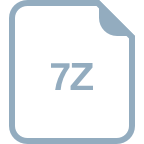
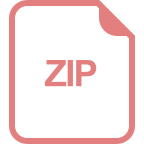
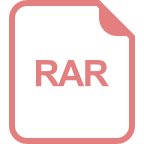
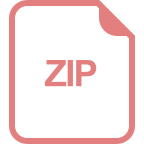
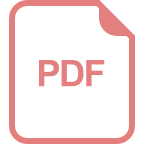
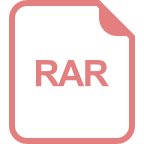
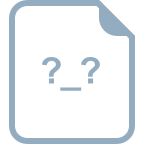
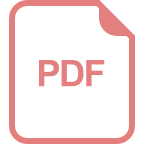
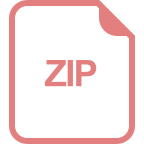
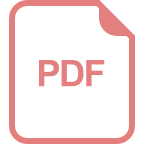
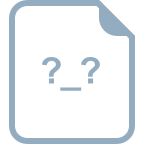
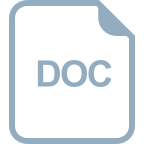
