单链表输入输出查找第i个元素主函数代码
时间: 2023-05-30 19:04:56 浏览: 59
#include <stdio.h>
#include <stdlib.h>
// 定义链表结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 插入节点
void insert(Node** head, int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
if (*head == NULL) {
*head = newNode;
return;
}
Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
// 查找第i个元素
Node* find(Node* head, int i) {
int count = 0;
Node* current = head;
while (current != NULL) {
count++;
if (count == i) {
return current;
}
current = current->next;
}
return NULL;
}
// 输出链表
void printList(Node* head) {
Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
}
int main() {
Node* head = NULL;
int data, i;
// 输入链表
printf("输入链表元素,以-1结束:\n");
scanf("%d", &data);
while (data != -1) {
insert(&head, data);
scanf("%d", &data);
}
// 输出链表
printf("链表元素为:");
printList(head);
printf("\n");
// 查找第i个元素
printf("输入要查找的元素的位置i:");
scanf("%d", &i);
Node* node = find(head, i);
if (node == NULL) {
printf("未找到第%d个元素\n", i);
} else {
printf("第%d个元素为:%d\n", i, node->data);
}
return 0;
}
相关推荐
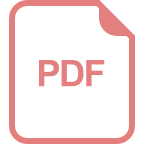
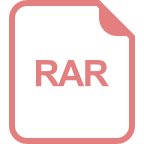
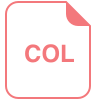














