使用RS服务作为后端设计一个玩家积分管理系统(服务后端+jsf 前端)
时间: 2024-06-09 18:09:06 浏览: 124
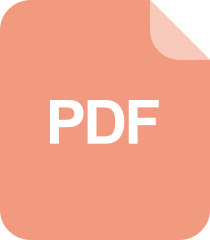
一个客户积分管理系统的设计与实现
好的,我来为您介绍一下如何使用RS服务设计一个玩家积分管理系统。
首先,我们需要定义玩家积分的数据结构,这里我们可以使用Java类来表示:
```java
public class PlayerScore {
private String playerName;
private int score;
public PlayerScore(String playerName, int score) {
this.playerName = playerName;
this.score = score;
}
// getter and setter methods
}
```
接下来,我们需要创建一个RS服务,用于管理玩家积分。这里我们使用Spring Boot框架来创建服务。首先,在`pom.xml`文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-rest</artifactId>
</dependency>
```
然后,创建一个`PlayerScoreRepository`接口,用于操作玩家积分数据:
```java
@RepositoryRestResource(collectionResourceRel = "player_scores", path = "player_scores")
public interface PlayerScoreRepository extends JpaRepository<PlayerScore, Long> {
List<PlayerScore> findByPlayerName(@Param("name") String name);
}
```
在上面的代码中,我们使用了`@RepositoryRestResource`注解来将`PlayerScoreRepository`暴露为RS服务,并定义了服务的URL路径为`/player_scores`。`findByPlayerName`方法用于根据玩家名称查询积分数据。
接下来,我们需要创建一个前端页面来管理玩家积分。这里我们使用JSF框架来创建页面。首先,在`pom.xml`文件中添加以下依赖:
```xml
<dependency>
<groupId>javax.faces</groupId>
<artifactId>javax.faces-api</artifactId>
<version>2.3</version>
</dependency>
<dependency>
<groupId>org.glassfish</groupId>
<artifactId>javax.faces</artifactId>
<version>2.3.0</version>
</dependency>
```
然后,在`webapp`目录下创建一个`index.xhtml`页面:
```html
<!DOCTYPE html>
<html lang="en"
xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html"
xmlns:f="http://xmlns.jcp.org/jsf/core">
<h:head>
<title>Player Score Management</title>
</h:head>
<h:body>
<h1>Player Score Management</h1>
<h:form>
<h:panelGrid columns="2">
<h:outputLabel for="playerName" value="Player Name:"/>
<h:inputText id="playerName" value="#{playerScoreBean.playerName}"/>
<h:outputLabel for="score" value="Score:"/>
<h:inputText id="score" value="#{playerScoreBean.score}"/>
<h:commandButton value="Add" action="#{playerScoreBean.addPlayerScore()}"/>
</h:panelGrid>
</h:form>
<h:form>
<h:panelGrid columns="2">
<h:outputLabel for="searchName" value="Search by Name:"/>
<h:inputText id="searchName" value="#{playerScoreBean.searchName}"/>
<h:commandButton value="Search" action="#{playerScoreBean.searchPlayerScore()}"/>
</h:panelGrid>
</h:form>
<h:dataTable value="#{playerScoreBean.playerScores}" var="playerScore">
<h:column>
<f:facet name="header">Player Name</f:facet>
#{playerScore.playerName}
</h:column>
<h:column>
<f:facet name="header">Score</f:facet>
#{playerScore.score}
</h:column>
</h:dataTable>
</h:body>
</html>
```
在上面的代码中,我们使用了JSF标签来创建一个简单的页面,包括添加玩家积分、查询玩家积分和展示玩家积分列表功能。这个页面使用了一个`PlayerScoreBean`来处理与后端RS服务的交互。
```java
@ManagedBean
@RequestScoped
public class PlayerScoreBean {
private String playerName;
private int score;
private String searchName;
private List<PlayerScore> playerScores;
public void addPlayerScore() {
RestTemplate restTemplate = new RestTemplate();
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
PlayerScore playerScore = new PlayerScore(playerName, score);
HttpEntity<PlayerScore> request = new HttpEntity<>(playerScore, headers);
restTemplate.postForObject("http://localhost:8080/player_scores", request, PlayerScore.class);
playerName = null;
score = 0;
searchName = null;
playerScores = null;
}
public void searchPlayerScore() {
RestTemplate restTemplate = new RestTemplate();
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
String url = "http://localhost:8080/player_scores/search/findByPlayerName?name=" + searchName;
HttpEntity<String> request = new HttpEntity<>(headers);
ResponseEntity<List<PlayerScore>> response = restTemplate.exchange(url, HttpMethod.GET, request, new ParameterizedTypeReference<List<PlayerScore>>() {});
playerScores = response.getBody();
searchName = null;
}
// getter and setter methods
}
```
在上面的代码中,`PlayerScoreBean`定义了添加玩家积分和查询玩家积分的方法,并使用`RestTemplate`类与后端RS服务进行交互。
最后,我们还需要创建一个`Application`类来启动RS服务:
```java
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
好了,现在你已经学会了如何使用RS服务和JSF框架来创建一个玩家积分管理系统,快来试试吧!
阅读全文
相关推荐
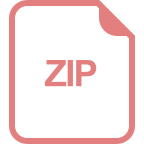

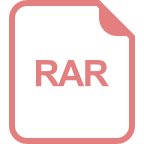
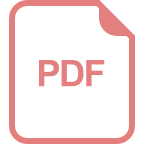
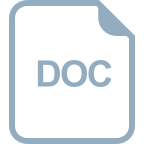
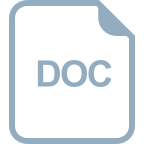
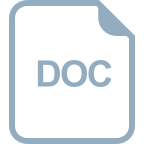
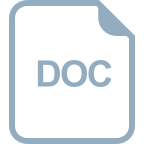
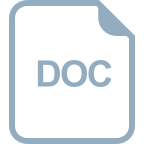
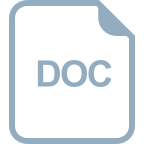
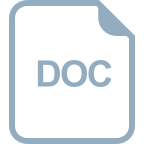
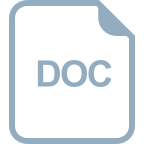
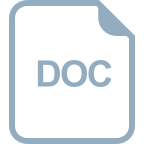
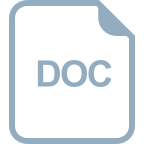
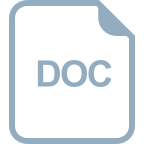
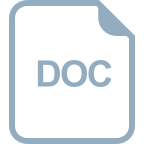
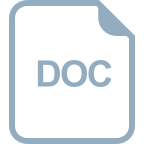