springgboot+vue实现下载Excel功能
时间: 2023-10-23 09:14:18 浏览: 45
要实现Spring Boot + Vue下载Excel功能,需要完成以下步骤:
1. 在后端(Spring Boot)中,使用Apache POI或其他Excel生成工具将数据转换为Excel格式。
2. 在Controller中,添加一个接口用于下载Excel文件。例如:
```java
@GetMapping("/downloadExcel")
public ResponseEntity<byte[]> downloadExcel() throws IOException {
// 生成Excel文件
File file = excelService.generateExcel();
// 设置响应头
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
headers.setContentDispositionFormData("attachment", "data.xlsx");
// 返回Excel文件的字节数组
return new ResponseEntity<>(FileUtils.readFileToByteArray(file), headers, HttpStatus.OK);
}
```
3. 在前端(Vue)中,添加一个按钮或链接,用于触发下载Excel文件的接口。例如:
```html
<template>
<div>
<button @click="downloadExcel">下载Excel</button>
</div>
</template>
<script>
import axios from 'axios'
export default {
methods: {
downloadExcel() {
axios({
url: '/downloadExcel',
method: 'GET',
responseType: 'blob'
}).then(response => {
const url = window.URL.createObjectURL(new Blob([response.data]))
const link = document.createElement('a')
link.href = url
link.setAttribute('download', 'data.xlsx')
document.body.appendChild(link)
link.click()
})
}
}
}
</script>
```
在这个例子中,我们使用axios库发送GET请求,响应类型为blob。之后,我们将响应的二进制数据转换为URL,并创建一个链接,模拟用户点击该链接,从而下载Excel文件。
这就是Spring Boot + Vue下载Excel文件的基本流程。当然,具体实现还需要根据自己的项目需求进行调整。
相关推荐
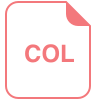













