一个页面做分页,每一页有12个卡片,头部有全选,卡片中有复选框,点击全选选中并保存id数组,翻页有保留选中状态,全选成半状态,帮我用vue3+element实现以上代码
时间: 2024-01-12 09:05:38 浏览: 178
好的,针对您的需求,我为您提供一个基于Vue3和Element UI的示例代码。您可以参考下面的代码来实现您的功能:
```vue
<template>
<div>
<!-- 全选框 -->
<el-checkbox v-model="isAllChecked" @change="handleAllCheckedChange" :indeterminate="isIndeterminate">
{{ isAllChecked ? '已全选' : '全选' }}
</el-checkbox>
<!-- 卡片列表 -->
<el-row>
<el-col v-for="card in currentCards" :key="card.id" :span="6">
<el-card>
<el-checkbox v-model="checkedIds" :label="card.id" @change="handleCardCheckedChange(card.id)">
{{ card.name }}
</el-checkbox>
</el-card>
</el-col>
</el-row>
<!-- 分页器 -->
<el-pagination :current-page="currentPage" :page-size="pageSize" :total="totalCards" @current-change="handlePageChange" />
</div>
</template>
<script>
import { ref, computed, watch } from 'vue'
export default {
name: 'MyPage',
data() {
return {
cards: [], // 所有卡片
checkedIds: [], // 已选中的卡片id数组
isAllChecked: false, // 是否已全选
isIndeterminate: false, // 是否半选
currentPage: 1, // 当前页码
pageSize: 12, // 每页显示数量
totalCards: 0, // 总共的卡片数量
}
},
computed: {
// 当前页的卡片列表
currentCards() {
const start = (this.currentPage - 1) * this.pageSize
const end = this.currentPage * this.pageSize
return this.cards.slice(start, end)
},
},
methods: {
// 全选框变化时的处理函数
handleAllCheckedChange(value) {
this.isAllChecked = value
this.checkedIds = value ? this.cards.map(card => card.id) : []
},
// 卡片复选框变化时的处理函数
handleCardCheckedChange(id) {
if (this.checkedIds.includes(id)) {
// 取消选中
this.checkedIds.splice(this.checkedIds.indexOf(id), 1)
this.isAllChecked = false
} else {
// 选中
this.checkedIds.push(id)
if (this.checkedIds.length === this.currentCards.length) {
this.isAllChecked = true
}
}
},
// 页面变化时的处理函数
handlePageChange(page) {
this.currentPage = page
},
},
watch: {
// 监听已选中的卡片id数组变化,计算全选框状态和是否半选
checkedIds: {
handler(value) {
if (value.length === 0) {
this.isAllChecked = false
this.isIndeterminate = false
} else if (value.length === this.currentCards.length) {
this.isAllChecked = true
this.isIndeterminate = false
} else {
this.isAllChecked = false
this.isIndeterminate = true
}
},
immediate: true,
},
// 监听currentPage变化,重新计算当前页的卡片列表
currentPage: {
handler(value) {
const start = (value - 1) * this.pageSize
const end = value * this.pageSize
this.currentCards = this.cards.slice(start, end)
},
immediate: true,
},
},
created() {
// 模拟异步获取卡片数据
setTimeout(() => {
this.cards = [
{ id: 1, name: '卡片1' },
{ id: 2, name: '卡片2' },
{ id: 3, name: '卡片3' },
{ id: 4, name: '卡片4' },
{ id: 5, name: '卡片5' },
{ id: 6, name: '卡片6' },
{ id: 7, name: '卡片7' },
{ id: 8, name: '卡片8' },
{ id: 9, name: '卡片9' },
{ id: 10, name: '卡片10' },
{ id: 11, name: '卡片11' },
{ id: 12, name: '卡片12' },
{ id: 13, name: '卡片13' },
{ id: 14, name: '卡片14' },
{ id: 15, name: '卡片15' },
{ id: 16, name: '卡片16' },
{ id: 17, name: '卡片17' },
{ id: 18, name: '卡片18' },
{ id: 19, name: '卡片19' },
{ id: 20, name: '卡片20' },
{ id: 21, name: '卡片21' },
{ id: 22, name: '卡片22' },
{ id: 23, name: '卡片23' },
{ id: 24, name: '卡片24' },
{ id: 25, name: '卡片25' },
]
this.totalCards = this.cards.length
}, 1000)
},
}
</script>
```
以上代码中,我们使用了Element UI的组件来实现全选框、卡片列表和分页器。通过计算属性和监听器,我们实现了卡片列表的分页、全选和半选的状态切换,以及保留已选中卡片状态的功能。同时,我们使用了Vue3的Composition API来组织代码,使其更加清晰易懂。
希望以上代码能够对您有所帮助。如果您还有其他问题或需要进一步的帮助,请随时问我。
阅读全文
相关推荐
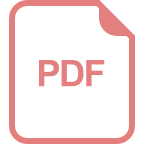
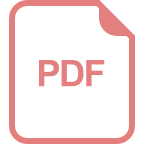


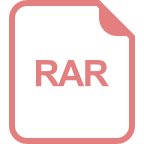
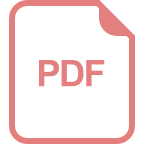
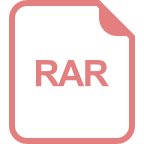
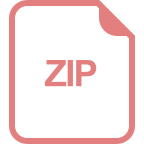
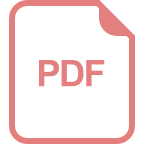
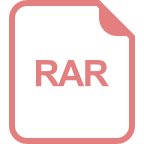
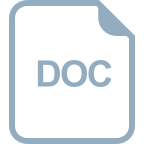
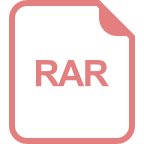
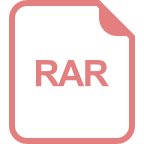
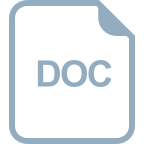



