c#启动exe 多种方式
时间: 2023-07-15 09:14:44 浏览: 61
在 C# 中启动一个外部的 EXE 文件,有多种方式可以实现。下面介绍几种常用的方法:
1. 使用 Process.Start 方法启动 EXE 文件
```csharp
using System.Diagnostics;
Process.Start("path/to/exe/file.exe");
```
2. 使用 ProcessStartInfo 类来配置启动参数
```csharp
using System.Diagnostics;
var startInfo = new ProcessStartInfo
{
FileName = "path/to/exe/file.exe",
Arguments = "/arg1 /arg2",
WorkingDirectory = "path/to/working/directory"
};
Process.Start(startInfo);
```
3. 使用 ShellExecuteEx 函数启动 EXE 文件
```csharp
using System.Runtime.InteropServices;
[StructLayout(LayoutKind.Sequential)]
public struct SHELLEXECUTEINFO
{
public int cbSize;
public uint fMask;
public IntPtr hwnd;
[MarshalAs(UnmanagedType.LPTStr)]
public string lpVerb;
[MarshalAs(UnmanagedType.LPTStr)]
public string lpFile;
[MarshalAs(UnmanagedType.LPTStr)]
public string lpParameters;
[MarshalAs(UnmanagedType.LPTStr)]
public string lpDirectory;
public int nShow;
public IntPtr hInstApp;
public IntPtr lpIDList;
[MarshalAs(UnmanagedType.LPTStr)]
public string lpClass;
public IntPtr hkeyClass;
public uint dwHotKey;
public IntPtr hIcon;
public IntPtr hProcess;
}
[DllImport("shell32.dll", SetLastError = true)]
static extern bool ShellExecuteEx(ref SHELLEXECUTEINFO lpExecInfo);
private void StartExe(string filePath, string arguments)
{
var startInfo = new SHELLEXECUTEINFO
{
cbSize = Marshal.SizeOf(typeof(SHELLEXECUTEINFO)),
lpFile = filePath,
lpParameters = arguments,
nShow = 1
};
ShellExecuteEx(ref startInfo);
}
```
以上是三种常用的启动外部 EXE 文件的方式,你可以根据自己的需要选择合适的方法。
相关推荐
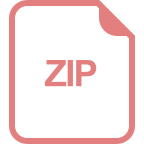
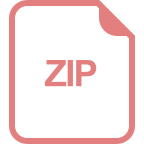
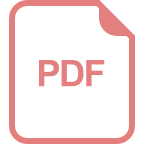














