Image.fromarray().show()
时间: 2023-10-16 11:14:18 浏览: 76
这是一个Python中PIL库的方法,用于将Numpy数组转换为图像并显示。具体使用方法如下:
```python
from PIL import Image
import numpy as np
# 创建一个随机的3通道图像
img_array = np.random.randint(0, 255, (300, 300, 3)).astype('uint8')
# 将数组转换为图像并显示
img = Image.fromarray(img_array)
img.show()
```
这将显示一个300x300像素的随机图像。注意,在使用`show()`方法之前,必须先安装好PIL库,并且需要在本地环境中打开图像查看器来显示图像。
相关问题
Image.fromarray
`Image.fromarray` 是 Python PIL (Python Imaging Library) 库中的一个函数,可以将一个 Numpy 数组转换为 PIL Image 对象。这个函数在将图像数据从 Numpy 数组转换为 PIL 图像对象时非常有用。例如,如果您有一个 Numpy 数组表示图像,您可以使用 `Image.fromarray` 将其转换为 PIL 图像对象,并进行一些图像处理和分析。
以下是一个示例代码:
```python
import numpy as np
from PIL import Image
# 创建一个随机的 numpy 数组表示图像
img_np = np.random.rand(256, 256, 3) * 255
img_np = img_np.astype(np.uint8)
# 将 numpy 数组转换为 PIL 图像对象
img_pil = Image.fromarray(img_np)
# 显示 PIL 图像对象
img_pil.show()
```
在上面的代码中,我们首先创建了一个随机的 numpy 数组表示图像,然后使用 `Image.fromarray` 将其转换为 PIL 图像对象,并最终显示了该图像。
Image.fromarray函数
`Image.fromarray` 是一个在Python中使用的函数,它属于PIL(Python Imaging Library)库的一部分,或者更准确地说,它属于这个库的后继者Pillow库中的功能。该函数用于将一个数值数组转换成Pillow库可以处理的图像对象。
Pillow是PIL的一个活跃分支,它为图像操作提供了大量功能,包括创建、操作和保存多种格式的图像。使用`Image.fromarray`函数时,你需要先创建一个数值数组,通常这个数组是二维的,并且包含了图像的像素数据。然后,你可以通过`Image.fromarray`将这个数组转换成一个Pillow的Image对象,之后就可以使用Pillow提供的各种图像处理功能了。
这个函数的典型使用流程如下:
1. 导入Pillow库中的Image模块。
2. 创建或获取一个合适的二维数值数组,该数组中的每个数值代表图像的一个像素点。
3. 使用`Image.fromarray`函数将数组转换为Image对象。
4. 利用Pillow提供的方法对Image对象进行操作。
5. 如果需要,还可以将Image对象保存为文件。
举个简单的例子,假设你有一个表示灰度图像的二维NumPy数组`array`,你可以这样做:
```python
from PIL import Image
import numpy as np
# 假设array是一个灰度图像的二维数组
image = Image.fromarray(array)
image.show()
```
或者如果数组是彩色图像的,通常是一个三维数组(高度、宽度、颜色通道),则需要先确保数据格式正确:
```python
# 假设array是一个彩色图像的三维数组
image = Image.fromarray(np.uint8(array))
image.show()
```
阅读全文
相关推荐
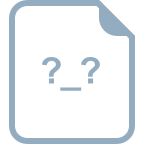
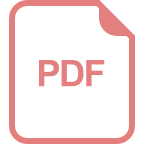
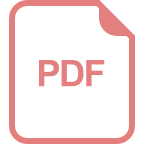











