使用Csharpe编写像素小鸟小游戏
时间: 2023-07-11 09:46:15 浏览: 198
以下是使用 C# 和 Unity 引擎编写的像素小鸟小游戏代码:
1. 创建一个新的 Unity 项目,并创建一个 2D 场景。
2. 将游戏资源文件(包括背景、小鸟、水管和游戏结束的图片)导入到 Unity 项目中,并将其放置在场景中。
3. 创建一个新的 C# 脚本,命名为 "BirdController",并将其附加到小鸟游戏对象上。代码如下:
```csharp
using UnityEngine;
public class BirdController : MonoBehaviour
{
public float jumpForce = 200f;
public float gravity = 2f;
public AudioClip jumpSound;
public AudioClip dieSound;
private Rigidbody2D rb;
private Animator anim;
private AudioSource audioSource;
private bool isDead = false;
void Start()
{
rb = GetComponent<Rigidbody2D>();
anim = GetComponent<Animator>();
audioSource = GetComponent<AudioSource>();
}
void Update()
{
if (Input.GetKeyDown(KeyCode.Space) && !isDead)
{
Jump();
}
ApplyGravity();
CheckDead();
}
void Jump()
{
rb.velocity = Vector2.zero;
rb.AddForce(new Vector2(0, jumpForce));
anim.SetTrigger("Flap");
audioSource.PlayOneShot(jumpSound);
}
void ApplyGravity()
{
rb.AddForce(new Vector2(0, -gravity));
}
void CheckDead()
{
if (!isDead && transform.position.y < -5.5f)
{
isDead = true;
anim.SetTrigger("Die");
audioSource.PlayOneShot(dieSound);
GameController.Instance.GameOver();
}
}
void OnCollisionEnter2D(Collision2D collision)
{
if (!isDead)
{
isDead = true;
anim.SetTrigger("Die");
audioSource.PlayOneShot(dieSound);
GameController.Instance.GameOver();
}
}
}
```
4. 创建一个新的 C# 脚本,命名为 "GameController",并将其附加到一个空对象上。代码如下:
```csharp
using UnityEngine;
using UnityEngine.SceneManagement;
using UnityEngine.UI;
public class GameController : MonoBehaviour
{
public static GameController Instance;
public GameObject pipePrefab;
public float pipeSpawnInterval = 2f;
public float pipeSpeed = -2f;
public Text scoreText;
public GameObject gameOverPanel;
private int score = 0;
private float pipeSpawnTimer = 0f;
void Awake()
{
if (Instance == null)
{
Instance = this;
}
}
void Start()
{
InvokeRepeating("SpawnPipe", 0f, pipeSpawnInterval);
}
void Update()
{
pipeSpawnTimer += Time.deltaTime;
}
void SpawnPipe()
{
if (pipeSpawnTimer >= pipeSpawnInterval)
{
float y = Random.Range(-1.8f, 2.3f);
GameObject pipe = Instantiate(pipePrefab);
pipe.transform.position = new Vector2(4.5f, y);
pipe.GetComponent<Rigidbody2D>().velocity = new Vector2(pipeSpeed, 0f);
pipeSpawnTimer = 0f;
}
}
public void AddScore()
{
score++;
scoreText.text = score.ToString();
}
public void GameOver()
{
gameOverPanel.SetActive(true);
}
public void Restart()
{
SceneManager.LoadScene(SceneManager.GetActiveScene().buildIndex);
}
}
```
5. 在 Unity 编辑器中,将 "BirdController" 脚本中的 jumpSound 和 dieSound 字段分别设置为跳跃音效和游戏结束音效的 AudioClip。
6. 在 Unity 编辑器中,将 "GameController" 脚本中的 pipePrefab 字段设置为水管的预制体,并将 scoreText 字段设置为显示分数的 Text 组件。
7. 在 Unity 编辑器中,将游戏结束的图片添加到一个新的 UI Panel 中,并将其设置为不可见状态。
8. 在 Unity 编辑器中,将 "GameController" 脚本中的 gameOverPanel 字段设置为上一步中的 UI Panel。
9. 在 Unity 编辑器中,创建一个 UI Button,命名为 "RestartButton",并将其添加到游戏结束的 UI Panel 中。
10. 在 Unity 编辑器中,将 "GameController" 脚本中的 Restart 方法分配给 "RestartButton" 的 onClick 事件。
11. 在 Unity 编辑器中,运行游戏,并在场景中移动小鸟,以测试游戏是否正常工作。
注意:本代码仅供学习参考,请勿用于商业用途。
阅读全文
相关推荐
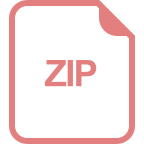
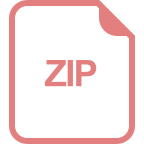
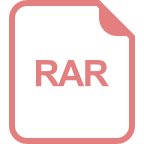
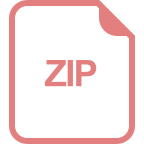
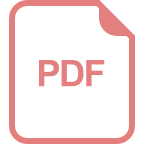
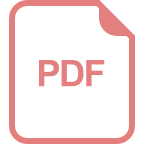
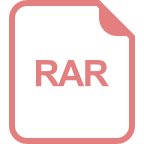
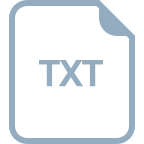
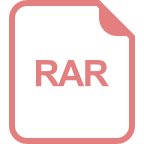
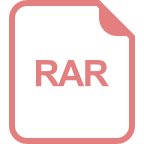
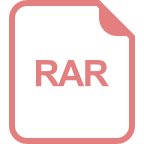
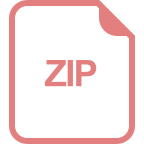
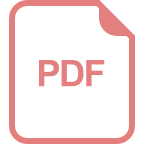
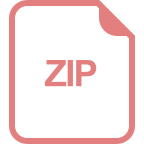


