S = require('crypto-js'); function _encryptPkcs7ToJava(e, t, n) { var o = S.enc.Utf8.parse(t) , r = S.enc.Utf8.parse(n) , a = S.enc.Utf8.parse(e); return S.AES.encrypt(a, o, { "iv": r, "mode": S.mode.CBC, "padding": S.pad.Pkcs7 }).ciphertext.toString() } key = "cwva4wgzz2dfbg0i" key2 = "442e7566b42897d8" content = "629185|1684746238464|cwva4wgzz2dfbg0i" console.log(_encryptPkcs7ToJava(content, key, key2)) 在python中ciphertext这个应该改成什么
时间: 2023-11-22 19:52:42 浏览: 37
在 Python 中可以使用 PyCryptodome 库来执行 AES 加密操作,将 `ciphertext` 改为 `encrypted_data`,代码如下:
```python
from Crypto.Cipher import AES
import base64
def _encryptPkcs7ToJava(e, t, n):
o = t.encode('utf-8')
r = n.encode('utf-8')
a = e.encode('utf-8')
cipher = AES.new(o, AES.MODE_CBC, r)
encrypted_data = cipher.encrypt(a)
return base64.b64encode(encrypted_data).decode('utf-8')
key = "cwva4wgzz2dfbg0i"
key2 = "442e7566b42897d8"
content = "629185|1684746238464|cwva4wgzz2dfbg0i"
print(_encryptPkcs7ToJava(content, key, key2))
```
注意,在 Python 中需要将字符串转换为字节串才能进行加密操作,因此需要使用 `encode()` 函数。同时,在 Python 中需要将加密后的结果进行 Base64 编码后输出,因此需要使用 `base64.b64encode()` 函数。
相关问题
bouncycastle的SM4加密js
BouncyCastle 是一个 Java 加密库,不过它也提供了 C#、C++、Python 和 JavaScript 等语言的支持。
以下是使用 BouncyCastle 实现 SM4 加密的 JavaScript 代码示例:
```javascript
// 引入 BouncyCastle 库
var CryptoJS = require('crypto-js');
var SM4Engine = require('bcrypto/lib/sm4');
// 定义 SM4 加密函数
function sm4Encrypt(key, plaintext) {
// 使用 BouncyCastle 实现 SM4 加密
var engine = new SM4Engine();
engine.init(true, key);
var input = CryptoJS.enc.Hex.parse(plaintext);
var output = CryptoJS.lib.WordArray.create(input.words.length, input.sigBytes);
engine.processBlock(input.words, 0, output.words, 0);
return CryptoJS.enc.Hex.stringify(output);
}
// 测试 SM4 加密函数
var key = CryptoJS.enc.Hex.parse('0123456789abcdeffedcba9876543210');
var plaintext = '0123456789abcdef';
var ciphertext = sm4Encrypt(key.words, plaintext);
console.log(ciphertext); // 输出:681edf34d206965e86b3e94f536e4246
```
需要注意的是,BouncyCastle 是一个 Java 加密库,因此需要使用一些工具将其转换为 JavaScript 可用的模块。在上面的示例中,我们使用了一个名为 `bcrypto` 的库来提供 BouncyCastle 的 JavaScript 实现。在使用时,您需要先安装 `crypto-js` 和 `bcrypto` 两个库:
```bash
npm install crypto-js bcrypto
```
然后就可以使用上面的代码示例来实现 SM4 加密了。
js逆向深圳信数据服务
根据提供的引用内容,似乎是在讨论对于一个加密的网址进行解密的问题。其中使用了AES加密算法和CryptoJS库来实现解密操作。具体代码如下:
const CryptoJS = require('crypto-js');
function getRealUrl(hh) {
var s = "qnbyzzwmdgghmcnm";
var aa = hh.split("/");
var aaa = aa.length;
var bbb = aa[aaa - 1].split('.');
var ccc = bbb;
var cccc = bbb;
var r = /^\ ?[1-9][0-9]*$/;
if (r.test(ccc) && cccc.indexOf('jhtml') != -1) {
var srcs = CryptoJS.enc.Utf8.parse(ccc);
var k = CryptoJS.enc.Utf8.parse(s);
var en = CryptoJS.AES.encrypt(srcs, k, { mode: CryptoJS.mode.ECB, padding: CryptoJS.pad.Pkcs7 });
var ddd = en.toString();
ddd = ddd.replace(/\//g, "^");
ddd = ddd.substring(0, ddd.length - 2);
var bbbb = ddd + '.' + bbb;
return bbbb;
}
}
getRealUrl()
这段代码是用于对加密的网址进行解密,具体的解密过程如下:
1. 将加密前的字符串cc分割成数组aa,获取数组长度aaa和数组最后一个元素bbb。
2. 判断最后一个元素bbb是否符合一定的格式要求。
3. 如果符合要求,则将cc转换成CryptoJS的Utf8格式的srcs,并生成一个Utf8格式的密钥k。
4. 使用AES加密算法将srcs和k进行加密操作,采用ECB模式和Pkcs7填充方式。
5. 将加密结果转换成字符串ddd,并替换其中的'/'字符为'^'。
6. 截取ddd中除去最后两个字符的部分,然后与bbb拼接起来,得到解密后的网址bbbb。
7. 返回解密后的网址bbbb。
关于js逆向深圳信数据服务的问题,根据提供的引用内容,目前并没有相关的信息来回答该问题。请提供更多相关的信息,以便更好地回答您的问题。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* [js逆向-某市公共资源交易网](https://blog.csdn.net/weixin_46672080/article/details/126003502)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *3* [aqistudy真气网JS逆向 + 数据采集(20220801)](https://blog.csdn.net/zhu6201976/article/details/126124548)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
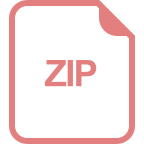
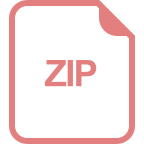
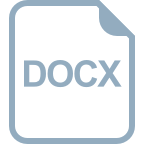




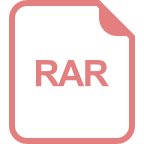
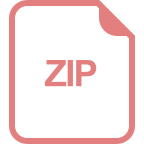
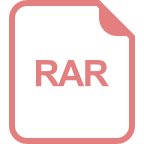
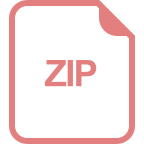
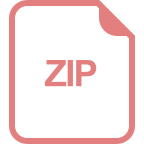
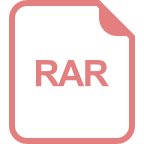