pytorch seq2seq+attention机器翻译
时间: 2023-10-21 19:06:43 浏览: 90
PyTorch是一种深度学习框架,可以用于实现序列到序列(seq2seq)的机器翻译任务。在seq2seq模型中,编码器将源序列编码为一个固定长度的向量,解码器则将该向量解码为目标序列。为了提高翻译质量,可以使用注意力机制来在解码器中引入上下文信息。
在PyTorch中实现seq2seq模型,可以使用nn.Module类来定义模型架构。首先,需要定义编码器和解码器的结构。编码器通常使用循环神经网络(RNN)或卷积神经网络(CNN)进行实现,而解码器则需要使用注意力机制。注意力机制可以使解码器关注输入序列中最相关的部分并根据其进行翻译。
实现注意力机制时,需要计算每个输入序列位置和当前解码器状态之间的相似度。这可以通过计算点积或使用神经网络来实现。然后,可以将相似度作为权重,对输入序列进行加权求和,以计算上下文向量。最后,将上下文向量与当前解码器状态组合在一起,以生成下一个目标序列符号的概率分布。
在训练过程中,可以使用交叉熵损失函数来计算模型输出与正确目标序列之间的差异,并使用反向传播算法更新模型参数。在推理过程中,可以使用贪婪搜索或束搜索来生成翻译结果。
总的来说,PyTorch提供了一种灵活且高效的方式来实现seq2seq模型和注意力机制,可以用于各种自然语言处理任务,包括机器翻译、问答系统和对话生成等。
相关问题
PyTorch中的Seq2Seq代码
在PyTorch中,Seq2Seq模型是一个常用的用于处理序列数据的模型,主要用于将一个序列转换为另一个序列。这种模型在自然语言处理、语音识别等领域都有广泛的应用。
Seq2Seq模型由两个部分组成:编码器和解码器。编码器将输入序列转换为一个固定大小的向量表示,解码器将这个向量表示转换为目标序列。在训练过程中,Seq2Seq模型会将编码器和解码器联合起来进行训练,以最小化输出序列与目标序列之间的差距。
在PyTorch中实现Seq2Seq模型通常需要使用nn.Module类来定义模型结构,同时还需要实现自定义的数据加载和预处理函数。在编码器中,通常使用RNN或CNN等结构对输入序列进行处理,并将最终的状态向量作为解码器的输入。在解码器中,通常使用RNN或Attention等机制来生成目标序列。
以下是一个简单的PyTorch Seq2Seq代码示例:
```
import torch
import torch.nn as nn
class Encoder(nn.Module):
def __init__(self, input_size, hidden_size):
super(Encoder, self).__init__()
self.hidden_size = hidden_size
self.embedding = nn.Embedding(input_size, hidden_size)
self.gru = nn.GRU(hidden_size, hidden_size)
def forward(self, input):
embedded = self.embedding(input)
output, hidden = self.gru(embedded)
return output, hidden
class Decoder(nn.Module):
def __init__(self, hidden_size, output_size):
super(Decoder, self).__init__()
self.hidden_size = hidden_size
self.embedding = nn.Embedding(output_size, hidden_size)
self.gru = nn.GRU(hidden_size, hidden_size)
self.out = nn.Linear(hidden_size, output_size)
self.softmax = nn.LogSoftmax(dim=1)
def forward(self, input, hidden):
output = self.embedding(input).view(1, 1, -1)
output, hidden = self.gru(output, hidden)
output = self.softmax(self.out(output))
return output, hidden
input_size = 100
output_size = 100
hidden_size = 256
encoder = Encoder(input_size, hidden_size)
decoder = Decoder(hidden_size, output_size)
criterion = nn.NLLLoss()
learning_rate = 0.01
encoder_optimizer = torch.optim.SGD(encoder.parameters(), lr=learning_rate)
decoder_optimizer = torch.optim.SGD(decoder.parameters(), lr=learning_rate)
for epoch in range(num_epochs):
for i, (input_seq, target_seq) in enumerate(data_loader):
encoder_optimizer.zero_grad()
decoder_optimizer.zero_grad()
input_len = input_seq.size(0)
target_len = target_seq.size(0)
encoder_hidden = torch.zeros(1, 1, hidden_size)
loss = 0
for j in range(input_len):
encoder_output, encoder_hidden = encoder(input_seq[j], encoder_hidden)
decoder_input = torch.tensor([[START_TOKEN]])
decoder_hidden = encoder_hidden
for j in range(target_len):
decoder_output, decoder_hidden = decoder(decoder_input, decoder_hidden)
loss += criterion(decoder_output, target_seq[j])
decoder_input = target_seq[j]
loss.backward()
encoder_optimizer.step()
decoder_optimizer.step()
```
seq2seq-attention 时间序列预测
seq2seq-attention模型是一种基于seq2seq模型的改进版本,用于时间序列预测。在seq2seq-attention模型中,引入了注意力机制,以便更好地处理长序列和提高预测准确性。
在seq2seq-attention模型中,输入序列首先通过编码器(Encoder)进行编码,得到一个固定长度的向量表示。编码器可以使用循环神经网络(RNN)或卷积神经网络(CNN)等结构。然后,解码器(Decoder)根据编码器的输出和之前的预测结果,逐步生成输出序列。
在每个解码步骤中,注意力机制被用来对编码器的输出进行加权,以便更关注与当前解码步骤相关的输入信息。这样可以提高模型对于长序列的处理能力,并且能够更好地捕捉输入序列中的重要特征。
总结来说,seq2seq-attention模型是一种用于时间序列预测的改进版本,通过引入注意力机制来提高模型的性能。它可以根据输入序列生成相应的输出序列,并且能够更好地处理长序列和捕捉重要特征。\[1\]\[2\]\[3\]
#### 引用[.reference_title]
- *1* *2* [PyTorch搭建LSTM实现多变量多步长时间序列预测(五):seq2seq](https://blog.csdn.net/Cyril_KI/article/details/125095225)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [NLP自然语言处理之RNN--LSTM--GRU--seq2seq--attention--self attetion](https://blog.csdn.net/weixin_41097516/article/details/103174768)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
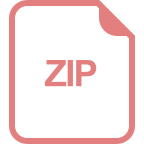
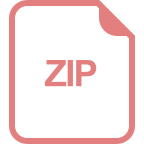












