msg.add_attachment
时间: 2023-12-14 19:04:13 浏览: 105
As an AI language model, I am not able to add attachments to messages. However, I can provide you with examples of how to add attachments in different programming languages:
In Python, you can use the `EmailMessage()` class from the `email` module to create and send an email message with attachments:
```python
import smtplib
from email.message import EmailMessage
msg = EmailMessage()
msg['Subject'] = 'Email with attachments'
msg['From'] = 'sender@example.com'
msg['To'] = 'recipient@example.com'
msg.set_content('This email contains attachments')
with open('file1.txt', 'rb') as f1, open('file2.txt', 'rb') as f2:
file1_data = f1.read()
file2_data = f2.read()
msg.add_attachment(file1_data, maintype='text', subtype='plain', filename='file1.txt')
msg.add_attachment(file2_data, maintype='text', subtype='plain', filename='file2.txt')
with smtplib.SMTP('smtp.gmail.com', 587) as smtp:
smtp.ehlo()
smtp.starttls()
smtp.login('sender@gmail.com', 'password')
smtp.send_message(msg)
```
In Java, you can use the JavaMail API to create and send an email message with attachments:
```java
import java.util.Properties;
import javax.activation.DataHandler;
import javax.activation.DataSource;
import javax.activation.FileDataSource;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeBodyPart;
import javax.mail.internet.MimeMessage;
import javax.mail.internet.MimeMultipart;
public class EmailExample {
public static void main(String[] args) {
String to = "recipient@example.com";
String from = "sender@example.com";
String host = "smtp.example.com";
Properties properties = System.getProperties();
properties.setProperty("mail.smtp.host", host);
Session session = Session.getDefaultInstance(properties);
try {
MimeMessage msg = new MimeMessage(session);
msg.setFrom(new InternetAddress(from));
msg.addRecipient(Message.RecipientType.TO, new InternetAddress(to));
msg.setSubject("Email with attachments");
MimeMultipart multipart = new MimeMultipart();
MimeBodyPart messageBodyPart = new MimeBodyPart();
messageBodyPart.setText("This email contains attachments");
multipart.addBodyPart(messageBodyPart);
messageBodyPart = new MimeBodyPart();
DataSource source = new FileDataSource("file1.txt");
messageBodyPart.setDataHandler(new DataHandler(source));
messageBodyPart.setFileName("file1.txt");
multipart.addBodyPart(messageBodyPart);
messageBodyPart = new MimeBodyPart();
source = new FileDataSource("file2.txt");
messageBodyPart.setDataHandler(new DataHandler(source));
messageBodyPart.setFileName("file2.txt");
multipart.addBodyPart(messageBodyPart);
msg.setContent(multipart);
Transport.send(msg);
System.out.println("Email sent successfully");
} catch (MessagingException mex) {
mex.printStackTrace();
}
}
}
```
Note: Please replace the placeholders with the actual values of your email credentials, file paths, etc.
阅读全文
相关推荐



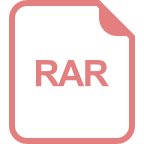



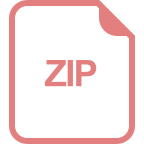
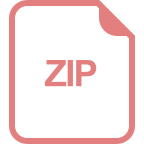
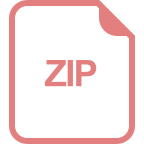



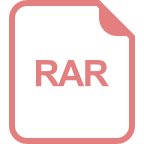
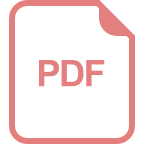
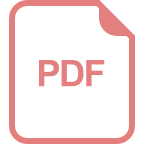