TypeScript 中使用yarn新建项目时修改 src/App.tsx,引入 antd 的按钮组件。
时间: 2024-03-23 21:36:52 浏览: 23
好的,首先需要确保安装了 TypeScript 和 yarn。然后,可以按照以下步骤来创建一个 TypeScript 项目并引入 antd 的按钮组件:
1. 在终端中进入项目存放的目录,使用以下命令创建一个新的 TypeScript 项目:
```
yarn create react-app my-app --template typescript
```
2. 进入项目目录:
```
cd my-app
```
3. 安装 antd:
```
yarn add antd
```
4. 打开 `src/App.tsx` 文件,并在顶部添加以下代码:
```typescript
import React from 'react';
import { Button } from 'antd';
import './App.css';
```
5. 在 `render` 方法中添加一个 `Button` 组件:
```typescript
function App() {
return (
<div className="App">
<Button type="primary">按钮</Button>
</div>
);
}
```
6. 运行项目:
```
yarn start
```
这样,你就可以在浏览器中看到一个带有 antd 按钮组件的页面了。
相关问题
TypeScript 中使用yarn新建项目时Command failed
如果在使用 TypeScript 和 Yarn 新建项目时遇到 Command failed,可能是因为缺少一些必要的依赖项或者 Yarn 版本过低导致的。以下是一些可能的解决方案:
1. 确认 Node.js 和 Yarn 的版本是否符合要求。Node.js 的版本应该大于等于 10.13,Yarn 的版本应该大于等于 1.5。
2. 尝试使用 npm 替代 Yarn 运行命令。
3. 如果使用了 Windows 操作系统,在运行命令前先以管理员身份运行命令提示符或 PowerShell。
4. 确认网络连接是否正常,有时候网络不稳定也会导致 Command failed。
5. 如果以上方法都没有解决问题,可以尝试卸载 Yarn 并重新安装。可以使用以下命令卸载 Yarn:
npm uninstall -g yarn
然后重新安装 Yarn:
npm install -g yarn
希望这些解决方案能够帮助你解决问题。如果还有其他问题,请随时提出。
TypeScript 中使用yarn新建项目
首先,确保你已经安装了 Node.js 和 yarn。
然后,按照以下步骤创建一个 TypeScript 项目:
1. 打开命令行窗口,进入你想要创建项目的目录。
2. 运行以下命令创建一个新的 TypeScript 项目:
```
yarn init -y
yarn add typescript --dev
```
3. 创建一个 `tsconfig.json` 文件,用来配置 TypeScript 编译器:
```
npx tsc --init
```
4. 在 `tsconfig.json` 文件中,将 `target` 字段设置为 `"es6"`,将 `outDir` 字段设置为 `"./dist"`。
5. 创建一个 `src` 目录,在其中创建一个 `index.ts` 文件,用来编写你的 TypeScript 代码。
6. 在 `package.json` 文件中,将 `scripts` 字段设置为如下所示:
```
"scripts": {
"build": "tsc",
"start": "node dist/index.js"
}
```
7. 运行以下命令编译 TypeScript 代码:
```
yarn build
```
现在,你可以运行以下命令启动项目:
```
yarn start
```
相关推荐
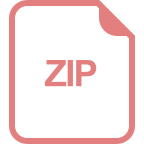
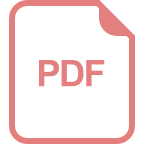
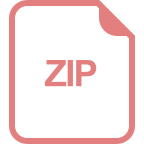













