你能在这段代码里面添加删除存储的数据功能package com.example.reciteword; import android.content.Context; import android.content.SharedPreferences; import android.os.Bundle; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.Button; import android.widget.ImageButton; import android.widget.ListView; import android.widget.TextView; import androidx.fragment.app.Fragment; import java.util.ArrayList; import java.util.List; import java.util.Random; public class wrongFragment extends Fragment { private List<Word> wordList = new ArrayList<>(); @Override public View onCreateView(LayoutInflater inflater, ViewGroup container,Bundle savedInstanceState) { return inflater.inflate(R.layout.fragment_wrong, container, false); } @Override public void onActivityCreated(Bundle savedInstanceState) { super.onActivityCreated(savedInstanceState); final SharedPreferences sharedPre = getActivity().getSharedPreferences("t",Context.MODE_PRIVATE); final int[] wrongNum = {sharedPre.getInt("wrongNum", 0)}; System.out.println(wrongNum[0]); wordList.clear(); for(int i = 1;i<=wrongNum[0];i++){ int temp = sharedPre.getInt("wrong"+i, 0); Word word = new Word(Data.getWord(temp),Data.getPron(temp),Data.getwordDefine(temp),sharedPre.getInt("word"+temp,1),0); System.out.println(Data.getRandNum()+":"+sharedPre.getInt("word"+temp,9)); wordList.add(word); } WordAdapter adapter = new WordAdapter(getActivity(),R.layout.word_item,wordList); ListView listView = (ListView) getActivity().findViewById(R.id.wrong_list_view); listView.setAdapter(adapter); } }
时间: 2023-06-16 14:05:19 浏览: 171
可以添加删除存储的数据功能,具体的实现方法如下:
1. 添加删除按钮
在布局文件fragment_wrong.xml中添加一个ImageButton按钮,用于删除存储的数据。代码如下:
```
<ImageButton
android:id="@+id/delete_btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="right"
android:src="@drawable/ic_delete" />
```
2. 实现删除功能
在onActivityCreated方法中获取删除按钮,并添加点击事件。当点击删除按钮时,遍历wordList中的所有单词,将其在SharedPreferences中存储的数据删除。具体代码如下:
```
ImageButton deleteBtn = getActivity().findViewById(R.id.delete_btn);
deleteBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
SharedPreferences.Editor editor = sharedPre.edit();
for (Word word : wordList) {
editor.remove("wrong" + word.getWordId());
editor.remove("word" + word.getWordId());
}
editor.putInt("wrongNum", 0);
editor.apply();
wordList.clear();
adapter.notifyDataSetChanged();
}
});
```
在删除单词时,需要将SharedPreferences中存储的单词总数wrongNum也同时清零,并且清空wordList中的所有单词。最后调用adapter的notifyDataSetChanged()方法,更新ListView的显示。
阅读全文
相关推荐
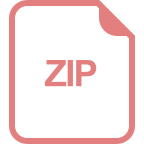
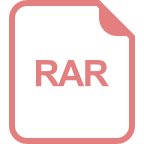

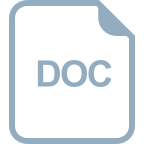
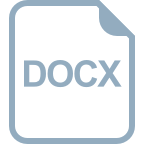
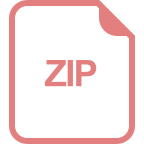
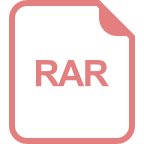
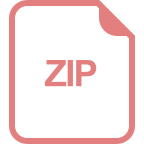