pyQT qprogressbar动态水波效果
时间: 2023-11-08 12:53:36 浏览: 57
要实现动态水波效果的QProgressBar,可以使用PyQt的QGraphicsView和QGraphicsScene类,以及第三方库pyqtgraph。
以下是一个使用pyqtgraph实现动态水波效果的QProgressBar的示例代码:
```python
from PyQt5.QtCore import QTimer
from PyQt5.QtGui import QBrush, QColor, QPalette
from PyQt5.QtWidgets import QApplication, QGraphicsView, QGraphicsScene, QWidget, QVBoxLayout
import pyqtgraph as pg
class ProgressBar(QWidget):
def __init__(self):
super().__init__()
layout = QVBoxLayout()
self.progress_bar = QProgressBarWave()
self.progress_bar.setRange(0, 100)
self.progress_bar.setTextVisible(False)
palette = QPalette()
palette.setColor(QPalette.Highlight, self.palette().color(QPalette.Active, QPalette.Highlight))
self.progress_bar.setPalette(palette)
layout.addWidget(self.progress_bar)
self.timer = QTimer()
self.timer.timeout.connect(self.update_progress)
self.timer.start(50)
self.setLayout(layout)
def update_progress(self):
value = self.progress_bar.value()
if value < 100:
self.progress_bar.setValue(value + 1)
else:
self.timer.stop()
self.progress_bar.setFormat("Done!")
class QProgressBarWave(QGraphicsView):
def __init__(self):
super().__init__()
self.scene = QGraphicsScene(self)
self.setScene(self.scene)
self.bar = pg.BarGraphItem(x=[0], height=[0], width=1, brush=QBrush(QColor(0, 120, 215)))
self.scene.addItem(self.bar)
self.wave = pg.PlotCurveItem(pen=pg.mkPen(QColor(0, 120, 215), width=2))
self.scene.addItem(self.wave)
def setValue(self, value):
self.value = value
self.update_bar()
def update_bar(self):
pos = [0, 1, 1, 0]
height = [self.value, self.value, 0, 0]
self.bar.setOpts(x=pos, height=height)
x = list(range(200))
y = [self.value * (1 + 0.3 * (i % 2) * (-1 if i % 4 < 2 else 1)) for i in range(200)]
self.wave.setData(x=x, y=y)
if __name__ == '__main__':
app = QApplication([])
window = ProgressBar()
window.show()
app.exec_()
```
在上面的示例中,我们创建了一个QProgressBarWave类,该类继承自QGraphicsView类,并使用QGraphicsScene和pyqtgraph库实现了动态水波效果的QProgressBar。在setValue()方法中,我们更新了进度值并调用update_bar()方法,该方法使用pg.BarGraphItem和pg.PlotCurveItem来更新进度条和水波效果。在ProgressBar类中,我们创建了一个QProgressBarWave对象,并使用QTimer在每50毫秒调用setValue()方法来更新进度值。
相关推荐
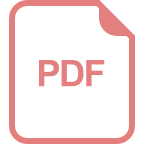
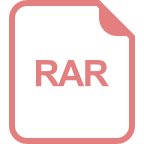














