.net .net framework 用多个声卡同时播放不同文本语音
时间: 2023-08-14 08:08:23 浏览: 177
在 .NET Framework 中,要同时使用多个声卡播放不同的文本语音,可以使用 DirectSound API 或者 Windows Multimedia API。下面是使用 Windows Multimedia API 的示例代码:
```
using System.Runtime.InteropServices;
using System.Threading;
using Microsoft.Win32.SafeHandles;
class Program
{
// 声明 Win32 API 函数
[DllImport("winmm.dll")]
private static extern int waveOutGetNumDevs();
[DllImport("winmm.dll")]
private static extern int waveOutOpen(out IntPtr hWaveOut, int uDeviceID, ref WaveFormat lpFormat, IntPtr dwCallback, IntPtr dwInstance, int dwFlags);
[DllImport("winmm.dll")]
private static extern int waveOutPrepareHeader(IntPtr hWaveOut, ref WaveHeader lpWaveOutHdr, int uSize);
[DllImport("winmm.dll")]
private static extern int waveOutWrite(IntPtr hWaveOut, ref WaveHeader lpWaveOutHdr, int uSize);
[DllImport("winmm.dll")]
private static extern int waveOutClose(IntPtr hWaveOut);
// 声明 WaveOutOpen 函数的参数结构体
[StructLayout(LayoutKind.Sequential)]
private struct WaveFormat
{
public short wFormatTag;
public short nChannels;
public int nSamplesPerSec;
public int nAvgBytesPerSec;
public short nBlockAlign;
public short wBitsPerSample;
public short cbSize;
}
// 声明 WaveOutPrepareHeader 函数的参数结构体
[StructLayout(LayoutKind.Sequential)]
private struct WaveHeader
{
public IntPtr lpData;
public int dwBufferLength;
public int dwBytesRecorded;
public IntPtr dwUser;
public int dwFlags;
public int dwLoops;
public IntPtr lpNext;
public IntPtr reserved;
}
static void Main(string[] args)
{
int numDevices = waveOutGetNumDevs();
if (numDevices < 2)
{
Console.WriteLine("没有足够的声卡!");
return;
}
// 打开第一和第二个声卡
IntPtr hWaveOut1, hWaveOut2;
WaveFormat format = new WaveFormat() { wFormatTag = 1, nChannels = 1, nSamplesPerSec = 8000, wBitsPerSample = 8, nBlockAlign = 1, nAvgBytesPerSec = 8000 };
waveOutOpen(out hWaveOut1, 0, ref format, IntPtr.Zero, IntPtr.Zero, 0);
waveOutOpen(out hWaveOut2, 1, ref format, IntPtr.Zero, IntPtr.Zero, 0);
// 打开两个语音文件
SafeFileHandle fileHandle1 = CreateFile("audio1.wav", FileAccess.Read, FileShare.Read, IntPtr.Zero, FileMode.Open, FileAttributes.Normal, IntPtr.Zero);
SafeFileHandle fileHandle2 = CreateFile("audio2.wav", FileAccess.Read, FileShare.Read, IntPtr.Zero, FileMode.Open, FileAttributes.Normal, IntPtr.Zero);
IntPtr hFile1 = fileHandle1.DangerousGetHandle();
IntPtr hFile2 = fileHandle2.DangerousGetHandle();
// 准备两个语音文件的缓冲区
const int BUFFER_SIZE = 4096;
byte[] buffer1 = new byte[BUFFER_SIZE];
byte[] buffer2 = new byte[BUFFER_SIZE];
var header1 = new WaveHeader() { lpData = Marshal.AllocHGlobal(BUFFER_SIZE), dwBufferLength = BUFFER_SIZE, dwFlags = 0 };
var header2 = new WaveHeader() { lpData = Marshal.AllocHGlobal(BUFFER_SIZE), dwBufferLength = BUFFER_SIZE, dwFlags = 0 };
int bytesRead1 = 0, bytesRead2 = 0;
ReadFile(hFile1, buffer1, BUFFER_SIZE, ref bytesRead1, IntPtr.Zero);
ReadFile(hFile2, buffer2, BUFFER_SIZE, ref bytesRead2, IntPtr.Zero);
Marshal.Copy(buffer1, 0, header1.lpData, bytesRead1);
Marshal.Copy(buffer2, 0, header2.lpData, bytesRead2);
waveOutPrepareHeader(hWaveOut1, ref header1, Marshal.SizeOf(typeof(WaveHeader)));
waveOutPrepareHeader(hWaveOut2, ref header2, Marshal.SizeOf(typeof(WaveHeader)));
// 开始播放两个语音文件
waveOutWrite(hWaveOut1, ref header1, Marshal.SizeOf(typeof(WaveHeader)));
waveOutWrite(hWaveOut2, ref header2, Marshal.SizeOf(typeof(WaveHeader)));
// 等待播放完成
while (header1.dwFlags != 1 || header2.dwFlags != 1)
{
Thread.Sleep(100);
}
// 关闭 WaveOut 和文件句柄
waveOutClose(hWaveOut1);
waveOutClose(hWaveOut2);
fileHandle1.Close();
fileHandle2.Close();
// 释放缓冲区内存
Marshal.FreeHGlobal(header1.lpData);
Marshal.FreeHGlobal(header2.lpData);
}
// 声明 CreateFile 函数,用于打开语音文件
[DllImport("kernel32.dll", CharSet = CharSet.Auto, SetLastError = true)]
private static extern SafeFileHandle CreateFile(
string lpFileName, FileAccess dwDesiredAccess, FileShare dwShareMode,
IntPtr lpSecurityAttributes, FileMode dwCreationDisposition,
FileAttributes dwFlagsAndAttributes, IntPtr hTemplateFile);
// 声明 ReadFile 函数,用于从语音文件中读取数据
[DllImport("kernel32.dll", SetLastError = true)]
private static extern bool ReadFile(IntPtr handle, byte[] bytes, int numBytesToRead, ref int numBytesRead, IntPtr overlapped);
}
```
上面的代码中,我们使用了 Windows Multimedia API 中的 WaveOutOpen、WaveOutPrepareHeader、WaveOutWrite 和 WaveOutClose 函数来播放语音文件。我们打开了两个声卡,并将两个语音文件分别设置到对应的声卡中。我们使用 SafeFileHandle 和 CreateFile 函数打开语音文件,并使用 ReadFile 函数从文件中读取数据,然后使用 WaveOutPrepareHeader 函数将数据设置到缓冲区中,最后使用 WaveOutWrite 函数播放缓冲区中的数据。我们在一个循环中等待语音文件播放完成,然后关闭相关的 WaveOut 和文件句柄,释放缓冲区内存。
阅读全文
相关推荐
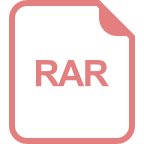








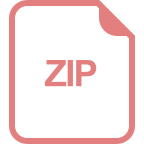
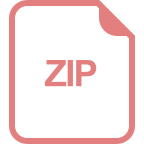
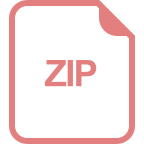
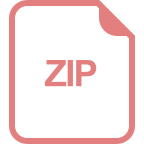