用链表管理学生信息。要求遍历链表,把所有学生的信息输出,学生的信息包含姓名,学号和三门成绩
时间: 2023-07-12 17:17:20 浏览: 48
好的,这是一个链表的经典应用。我可以给您提供一个基本的实现思路:
首先,我们需要定义一个学生结构体 `student`,包含姓名、学号和三门成绩的信息。同时,我们还需要定义一个链表结构体 `node`,包含一个指向学生结构体的指针 `stu` 和一个指向下一个节点的指针 `next`。
```
typedef struct student {
char name[20];
int id;
float score1;
float score2;
float score3;
} student;
typedef struct node {
student* stu;
struct node* next;
} node;
```
接下来,我们可以编写一个函数 `print_students`,该函数遍历链表并输出所有学生的信息。函数的实现思路如下:
1. 从链表的头节点开始遍历,直到遍历到最后一个节点为止;
2. 对于每一个节点,输出学生的姓名、学号和三门成绩的信息。
代码实现如下:
```
void print_students(node* head) {
node* p = head;
while (p != NULL) {
printf("Name: %s, ID: %d, Score1: %.2f, Score2: %.2f, Score3: %.2f\n",
p->stu->name, p->stu->id, p->stu->score1, p->stu->score2, p->stu->score3);
p = p->next;
}
}
```
最后,我们可以编写一个主函数,创建一个链表并向其中添加若干个学生的信息,然后调用 `print_students` 函数输出所有学生的信息。代码实现如下:
```
int main() {
// 创建头节点
node* head = (node*)malloc(sizeof(node));
head->stu = NULL;
head->next = NULL;
// 添加若干个学生
for (int i = 1; i <= 5; i++) {
student* stu = (student*)malloc(sizeof(student));
sprintf(stu->name, "Student%d", i);
stu->id = i;
stu->score1 = 80 + i * 5;
stu->score2 = 75 + i * 4;
stu->score3 = 90 + i * 3;
node* p = (node*)malloc(sizeof(node));
p->stu = stu;
p->next = NULL;
node* q = head;
while (q->next != NULL) {
q = q->next;
}
q->next = p;
}
// 输出所有学生的信息
print_students(head);
// 释放链表中的所有动态分配的内存
node* p = head;
while (p != NULL) {
node* q = p->next;
free(p->stu);
free(p);
p = q;
}
return 0;
}
```
希望这个实现思路可以帮助您解决问题。如果您还有任何疑问,请随时向我提出。
相关推荐
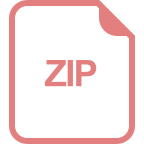
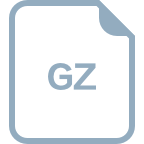














