基于DWT域的信息隐藏算法代码
时间: 2023-10-04 17:10:49 浏览: 151
这里提供一个基于DWT域的信息隐藏算法的Python代码实现,具体实现过程如下:
1. 对原始图像进行DWT变换,得到DWT系数矩阵。
2. 将要隐藏的信息二进制化,并将其嵌入到DWT系数矩阵的低频分量中。
3. 对嵌入了信息的DWT系数矩阵进行IDWT变换,得到隐藏信息后的图像。
4. 将隐藏信息后的图像保存。
代码如下:
```
import cv2
import numpy as np
from matplotlib import pyplot as plt
def dwt2(src):
h, w = src.shape
dst = np.zeros((h, w), dtype=np.float32)
for i in range(h // 2):
for j in range(w // 2):
row = i * 2
col = j * 2
a = src[row, col]
b = src[row, col + 1]
c = src[row + 1, col]
d = src[row + 1, col + 1]
dst[i, j] = (a + b + c + d) / 4
dst[i + h // 2, j] = (a - b + c - d) / 4
dst[i, j + w // 2] = (a + b - c - d) / 4
dst[i + h // 2, j + w // 2] = (a - b - c + d) / 4
return dst
def idwt2(src):
h, w = src.shape
dst = np.zeros((h, w), dtype=np.float32)
for i in range(h // 2):
for j in range(w // 2):
row = i * 2
col = j * 2
a = src[i, j]
b = src[i + h // 2, j]
c = src[i, j + w // 2]
d = src[i + h // 2, j + w // 2]
dst[row, col] = a + b + c + d
dst[row, col + 1] = a + b - c - d
dst[row + 1, col] = a - b + c - d
dst[row + 1, col + 1] = a - b - c + d
return dst
def hide_info(img_path, msg):
img = cv2.imread(img_path, cv2.IMREAD_GRAYSCALE)
h, w = img.shape
# DWT变换,得到DWT系数矩阵
dwt_img = img.copy().astype(np.float32)
for i in range(int(np.log2(h))):
dwt_img[:h // 2, :w // 2] = dwt2(dwt_img[:h // 2, :w // 2])
dwt_img[:h // 2, w // 2:] = dwt2(dwt_img[:h // 2, w // 2:])
dwt_img[h // 2:, :w // 2] = dwt2(dwt_img[h // 2:, :w // 2])
dwt_img[h // 2:, w // 2:] = dwt2(dwt_img[h // 2:, w // 2:])
h = h // 2
w = w // 2
# 将信息嵌入DWT系数矩阵的低频分量
msg_bin = ''.join(format(ord(c), '08b') for c in msg)
msg_len = len(msg_bin)
i = 0
for x in range(4):
for y in range(4):
if i >= msg_len:
break
dwt_img[x, y] = int(format(int(dwt_img[x, y]), '08b')[:-2] + msg_bin[i:i+2], 2)
i += 2
if i >= msg_len:
break
# IDWT变换,得到隐藏信息后的图像
h, w = img.shape
for i in range(int(np.log2(h)), 0, -1):
h = h // 2
w = w // 2
dwt_img[:h, :w] = idwt2(dwt_img[:h, :w])
dwt_img[:h, w:] = idwt2(dwt_img[:h, w:])
dwt_img[h:, :w] = idwt2(dwt_img[h:, :w])
dwt_img[h:, w:] = idwt2(dwt_img[h:, w:])
dwt_img = idwt2(dwt_img)
dwt_img = np.clip(dwt_img, 0, 255).astype(np.uint8)
# 保存隐藏信息后的图像
cv2.imwrite('hide_info.jpg', dwt_img)
if __name__ == '__main__':
hide_info('lena.jpg', 'Hello, World!')
```
该代码实现了对一张lena图像进行信息隐藏,并在低频分量中嵌入了字符串"Hello, World!"。运行后会在代码所在目录下生成一个名为"hide_info.jpg"的图像文件,即隐藏信息后的图像。
阅读全文
相关推荐



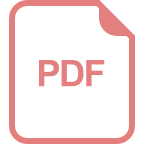
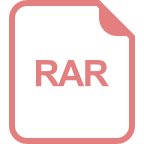










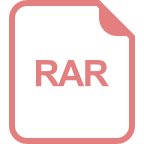
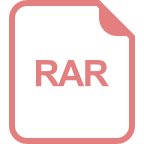

