学生评教系统 基本功能: 设计一个学生对老师的评教系统,基本功能包括:基本设置、查询,评教,统计。 一级菜单显示“1.基本设置 2.评教 3.统计 4.退出”。用户输入自己的选择后,进入相应的二级菜单。比如,选择“3”,则进入“统计”模块;当用户选择“4”时,退出系统。 基本设置包括设置(班级、学号、姓名),修改密码、(课程名、教师名称);并存入文件。 用户选择“2”时,开始评教,需要输入二级菜单显示“课程名,评教分数”,其中课程名由程序从事先设置好的文件读出,要求用户输入相应课程名对应的评教分数(百分制),输入完毕后,返回上一级菜单。 用户选择“2”时,系统可以将所有用户当前已评教的课程和分数全部显示出来,如: 拓展功能: 登录:在进入系统之前,要求用户输入用户名和密码,用户名是“manager”和密码是“111111”。用户可以通过基本设置里的“修改密码”选项来登陆密码。 用c语言来写
时间: 2023-12-26 20:03:36 浏览: 111
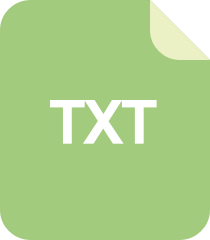
基本功能:设学生姓名及成绩已分别存入指定数据存储单元,统计成绩分布的人数:A级(90~100),B级(80~89),C级(70~79),D级(60~69),E级(0~59)。并按顺序显示全班成绩,及统计信息。要求界面友好。 增强功能:学生姓名及成绩根据屏幕提示由键盘录入再存入数据存储单元。

以下是一个简单的学生评教系统的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_COURSES 10 // 最大课程数
#define MAX_NAME_LEN 20 // 最大名称长度
struct Student {
char name[MAX_NAME_LEN];
char id[MAX_NAME_LEN];
char className[MAX_NAME_LEN];
};
struct Course {
char name[MAX_NAME_LEN];
char teacher[MAX_NAME_LEN];
};
struct Score {
char courseName[MAX_NAME_LEN];
int score;
};
struct User {
char name[MAX_NAME_LEN];
char password[MAX_NAME_LEN];
};
int menu(); // 一级菜单
void basicSettings(struct Student* student, struct Course courses[], int* numCourses); // 基本设置
void changePassword(struct User* user); // 修改密码
void evaluation(struct Course courses[], int numCourses, struct Score scores[], int* numScores); // 评教
void showScores(struct Score scores[], int numScores); // 查询评教分数
void statistics(struct Score scores[], int numScores); // 统计评教分数
int main() {
struct User user = {"manager", "111111"}; // 默认用户名和密码
struct Student student;
struct Course courses[MAX_COURSES];
int numCourses = 0;
struct Score scores[MAX_COURSES];
int numScores = 0;
char name[MAX_NAME_LEN];
char password[MAX_NAME_LEN];
printf("请输入用户名和密码:\n");
printf("用户名:");
scanf("%s", name);
printf("密码:");
scanf("%s", password);
if (strcmp(name, user.name) != 0 || strcmp(password, user.password) != 0) {
printf("用户名或密码错误,程序退出!\n");
return 0;
}
printf("欢迎使用学生评教系统!\n");
while (1) {
switch (menu()) {
case 1: basicSettings(&student, courses, &numCourses); break;
case 2: evaluation(courses, numCourses, scores, &numScores); break;
case 3: statistics(scores, numScores); break;
case 4: printf("谢谢使用,再见!\n"); return 0;
default: printf("无效的输入,请重新输入!\n");
}
}
return 0;
}
int menu() {
int choice;
printf("\n--------一级菜单--------\n");
printf("1.基本设置 2.评教 3.统计 4.退出\n");
printf("请输入您的选择:");
scanf("%d", &choice);
return choice;
}
void basicSettings(struct Student* student, struct Course courses[], int* numCourses) {
printf("\n--------基本设置--------\n");
printf("请输入班级:");
scanf("%s", student->className);
printf("请输入学号:");
scanf("%s", student->id);
printf("请输入姓名:");
scanf("%s", student->name);
printf("请输入课程数:");
scanf("%d", numCourses);
printf("请输入每门课程的名称和教师名字:\n");
for (int i = 0; i < *numCourses; i++) {
printf("第%d门课程:", i + 1);
scanf("%s %s", courses[i].name, courses[i].teacher);
}
FILE* fp = fopen("student.dat", "wb");
if (fp == NULL) {
printf("无法打开文件!\n");
return;
}
fwrite(student, sizeof(struct Student), 1, fp);
fclose(fp);
fp = fopen("courses.dat", "wb");
if (fp == NULL) {
printf("无法打开文件!\n");
return;
}
fwrite(courses, sizeof(struct Course), *numCourses, fp);
fclose(fp);
printf("设置已保存!\n");
}
void changePassword(struct User* user) {
printf("\n--------修改密码--------\n");
printf("请输入旧密码:");
char oldpassword[MAX_NAME_LEN];
scanf("%s", oldpassword);
if (strcmp(oldpassword, user->password) != 0) {
printf("密码错误,修改失败!\n");
return;
}
printf("请输入新密码:");
scanf("%s", user->password);
printf("密码已修改!\n");
}
void evaluation(struct Course courses[], int numCourses, struct Score scores[], int* numScores) {
printf("\n--------评教--------\n");
printf("请选择要评教的课程:\n");
for (int i = 0; i < numCourses; i++) {
printf("%d. %s\n", i + 1, courses[i].name);
}
int choice;
scanf("%d", &choice);
if (choice < 1 || choice > numCourses) {
printf("无效的选择!\n");
return;
}
printf("请输入评教分数(0-100):");
int score;
scanf("%d", &score);
if (score < 0 || score > 100) {
printf("无效的分数!\n");
return;
}
strcpy(scores[*numScores].courseName, courses[choice - 1].name);
scores[*numScores].score = score;
(*numScores)++;
printf("评教已完成!\n");
FILE* fp = fopen("scores.dat", "wb");
if (fp == NULL) {
printf("无法打开文件!\n");
return;
}
fwrite(scores, sizeof(struct Score), *numScores, fp);
fclose(fp);
}
void showScores(struct Score scores[], int numScores) {
printf("\n--------查询评教分数--------\n");
for (int i = 0; i < numScores; i++) {
printf("%s:%d\n", scores[i].courseName, scores[i].score);
}
}
void statistics(struct Score scores[], int numScores) {
printf("\n--------统计评教分数--------\n");
int total = 0;
for (int i = 0; i < numScores; i++) {
total += scores[i].score;
}
if (numScores != 0) {
printf("平均分数:%d\n", total / numScores);
}
else {
printf("还没有评教记录!\n");
}
}
```
这个程序使用了结构体来存储学生、课程、评教记录和用户信息。评教记录和课程信息会存储到文件中,以便下次程序运行时能够读取。评教分数采用了整数类型,范围为0到100。程序还提供了一个修改密码的功能,只有输入正确的旧密码才能修改密码。
阅读全文
相关推荐
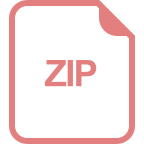
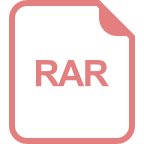
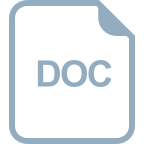
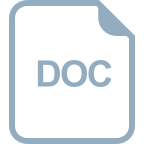
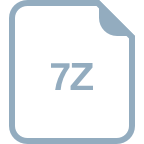
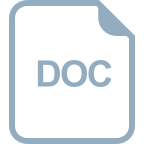
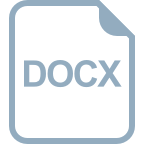
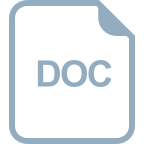
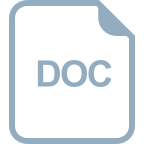
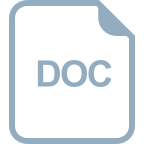
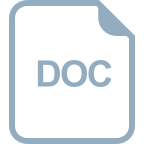
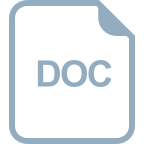
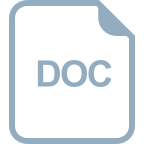
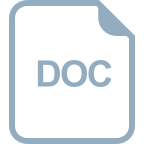
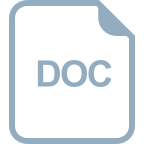
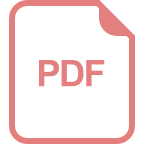
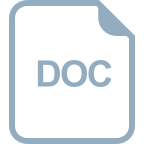
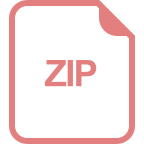