使用vue写一个带滚动条的表格
时间: 2024-05-01 13:16:55 浏览: 120
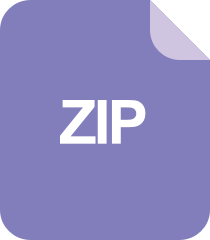
表格加滚动条
以下是一个使用Vue.js编写的带滚动条的表格示例:
HTML:
```html
<div id="app">
<div class="table-container">
<table class="table">
<thead>
<tr>
<th>序号</th>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in tableData" :key="index">
<td>{{index + 1}}</td>
<td>{{item.name}}</td>
<td>{{item.age}}</td>
<td>{{item.gender}}</td>
</tr>
</tbody>
</table>
<div class="scrollbar">
<div class="thumb" :style="{height: thumbHeight+'px', top: thumbTop+'px'}" @mousedown="startDrag"></div>
</div>
</div>
</div>
```
CSS:
```css
.table-container {
position: relative;
}
.table {
width: 100%;
border-collapse: collapse;
}
.table th,
.table td {
border: 1px solid #ccc;
padding: 8px;
text-align: center;
}
.scrollbar {
position: absolute;
right: 0;
top: 0;
bottom: 0;
width: 10px;
overflow: hidden;
background-color: #f5f5f5;
}
.thumb {
position: absolute;
left: 0;
width: 100%;
background-color: #ccc;
cursor: pointer;
}
```
JavaScript:
```javascript
new Vue({
el: '#app',
data: {
tableData: [
{ name: '张三', age: 18, gender: '男' },
{ name: '李四', age: 22, gender: '女' },
{ name: '王五', age: 30, gender: '男' },
{ name: '赵六', age: 25, gender: '女' },
{ name: '钱七', age: 28, gender: '男' },
{ name: '孙八', age: 20, gender: '女' },
{ name: '周九', age: 24, gender: '男' },
{ name: '吴十', age: 26, gender: '女' }
],
thumbTop: 0,
thumbHeight: 50,
dragStart: false,
startY: 0
},
computed: {
tableHeight() {
return this.$el.querySelector('.table-container').offsetHeight;
},
contentHeight() {
return this.$el.querySelector('tbody').offsetHeight;
},
scrollHeight() {
return this.tableHeight - this.contentHeight;
}
},
methods: {
startDrag(e) {
this.dragStart = true;
this.startY = e.clientY;
},
onDrag(e) {
if (this.dragStart) {
const deltaY = e.clientY - this.startY;
const newTop = this.thumbTop + deltaY;
if (newTop >= 0 && newTop <= this.scrollHeight) {
this.thumbTop = newTop;
const scrollPercent = this.thumbTop / this.scrollHeight;
this.$el.querySelector('tbody').style.transform = `translateY(-${scrollPercent * 100}%)`;
}
this.startY = e.clientY;
}
},
stopDrag() {
this.dragStart = false;
}
},
mounted() {
this.thumbHeight = this.tableHeight / this.contentHeight * this.tableHeight;
this.$el.querySelector('.scrollbar').addEventListener('mousemove', this.onDrag);
this.$el.querySelector('.scrollbar').addEventListener('mouseup', this.stopDrag);
},
beforeDestroy() {
this.$el.querySelector('.scrollbar').removeEventListener('mousemove', this.onDrag);
this.$el.querySelector('.scrollbar').removeEventListener('mouseup', this.stopDrag);
}
})
```
这个示例中,我们使用了CSS中的`position: absolute`将滚动条和表格分开,并使用计算属性和数据绑定来实现滚动条的拖动和表格内容的滚动。我们为滚动条的拖动区域绑定了`mousedown`事件,在鼠标按下时将`dragStart`设置为`true`,表示开始拖动。在`mousemove`事件中,如果`dragStart`为`true`,则计算拖动的距离,更新滚动条的位置和表格内容的偏移量。在`mouseup`事件中,将`dragStart`设置为`false`,表示停止拖动。最后,我们在组件的`mounted`和`beforeDestroy`钩子中分别添加和移除事件监听器。
阅读全文
相关推荐
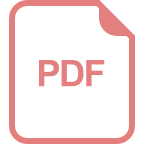












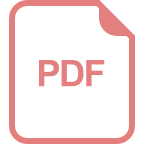