函数曲线的离散化图java
时间: 2023-06-13 18:05:17 浏览: 85
以下是一个简单的Java程序,用于将函数曲线离散化并绘制成图表。该程序使用了JFreeChart库来绘制图表。
```java
import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartFrame;
import org.jfree.chart.JFreeChart;
import org.jfree.chart.plot.PlotOrientation;
import org.jfree.data.xy.XYSeries;
import org.jfree.data.xy.XYSeriesCollection;
public class Discretization {
public static void main(String[] args) {
// 定义函数曲线
double[] xValues = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
double[] yValues = {0, 0.5, 1, 2.5, 3, 4, 3.5, 2, 1.5, 1, 0.5};
// 将函数曲线离散化
double[] xDiscrete = new double[101];
double[] yDiscrete = new double[101];
for (int i = 0; i <= 100; i++) {
double x = i * 0.1;
xDiscrete[i] = x;
yDiscrete[i] = interpolate(x, xValues, yValues);
}
// 绘制图表
XYSeriesCollection dataset = new XYSeriesCollection();
XYSeries series = new XYSeries("Function Curve");
for (int i = 0; i < xDiscrete.length; i++) {
series.add(xDiscrete[i], yDiscrete[i]);
}
dataset.addSeries(series);
JFreeChart chart = ChartFactory.createXYLineChart("Discretization", "x", "y",
dataset, PlotOrientation.VERTICAL, true, true, false);
ChartFrame frame = new ChartFrame("Discretization", chart);
frame.pack();
frame.setVisible(true);
}
private static double interpolate(double x, double[] xValues, double[] yValues) {
int i = 0;
while (i < xValues.length && xValues[i] < x) {
i++;
}
if (i == 0) {
return yValues[0];
} else if (i == xValues.length) {
return yValues[xValues.length - 1];
} else {
double x1 = xValues[i - 1];
double x2 = xValues[i];
double y1 = yValues[i - 1];
double y2 = yValues[i];
return y1 + (x - x1) / (x2 - x1) * (y2 - y1);
}
}
}
```
该程序定义了一个简单的函数曲线,并使用线性插值方法将其离散化为101个点。然后使用JFreeChart库创建了一个XY线图表,并将离散化后的函数曲线添加到数据集中。最后,该程序使用ChartFrame显示了图表。
你可以根据自己的需求修改函数曲线的定义和离散化方法,以及图表的设置。
相关推荐
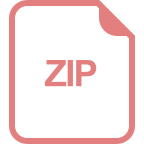
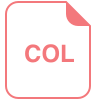
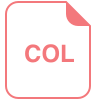












