使用python,编写基于遗传算法的步骤和服装产业的特点对车间作业调度问题进行数学建模。保存所需排程的订单号和产线号,关联订单在对应产线所需的生产时间,通过一维数组保存每个产线的最后生产时间,用另一个一维数组记录每个订单的开始作业时间和结束时间。
时间: 2024-03-10 08:43:36 浏览: 63
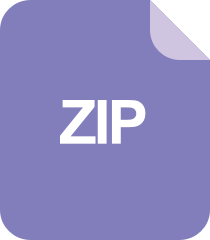
求解柔性作业车间调度问题 的遗传 算法实现_python_代码_下载

好的,让我来为你编写一个基于遗传算法的车间作业调度问题求解的Python代码。
首先,我们需要定义一个订单类来存储每个订单的相关信息,包括订单号、交货日期、所需生产时间和所在产线等。然后,我们可以定义一个调度类来实现遗传算法的各个操作,包括初始化种群、计算适应度、选择、交叉、变异等。最后,我们可以在主函数中调用调度类来求解最优的车间作业调度方案,并将结果保存到相应的数组中。
下面是一个简单的Python代码示例:
```python
import random
class Order:
def __init__(self, order_id, delivery_date, production_time, production_line):
self.order_id = order_id
self.delivery_date = delivery_date
self.production_time = production_time
self.production_line = production_line
class Schedule:
def __init__(self, orders, production_lines):
self.orders = orders
self.production_lines = production_lines
self.generation_size = 10
self.mutation_rate = 0.1
self.elite_rate = 0.2
def initialize_population(self):
population = []
for i in range(self.generation_size):
chromosome = random.sample(self.orders, len(self.orders))
population.append(chromosome)
return population
def calculate_fitness(self, chromosome):
production_times = [0] * len(self.production_lines)
start_times = [0] * len(chromosome)
end_times = [0] * len(chromosome)
for i in range(len(chromosome)):
order = chromosome[i]
production_line = order.production_line
production_time = order.production_time
start_time = max(production_times[production_line], start_times[i])
end_time = start_time + production_time
start_times[i] = start_time
end_times[i] = end_time
production_times[production_line] = end_time
fitness = max(production_times)
return fitness, start_times, end_times
def selection(self, population, fitnesses):
elite_size = int(self.generation_size * self.elite_rate)
elite_indices = sorted(range(len(fitnesses)), key=lambda k: fitnesses[k])[:elite_size]
elite_population = [population[i] for i in elite_indices]
selection_size = len(population) - elite_size
selection_indices = random.choices(range(len(population)), k=selection_size, weights=fitnesses)
selection_population = [population[i] for i in selection_indices]
return elite_population + selection_population
def crossover(self, parent1, parent2):
child = [None] * len(parent1)
start = random.randint(0, len(parent1) - 1)
end = random.randint(start, len(parent1) - 1)
for i in range(start, end + 1):
child[i] = parent1[i]
j = 0
for i in range(len(child)):
if child[i] is None:
while parent2[j] in child:
j += 1
child[i] = parent2[j]
j += 1
return child
def mutation(self, chromosome):
if random.random() < self.mutation_rate:
i = random.randint(0, len(chromosome) - 1)
j = random.randint(0, len(chromosome) - 1)
chromosome[i], chromosome[j] = chromosome[j], chromosome[i]
def evolve(self):
population = self.initialize_population()
for i in range(10):
fitnesses = []
for chromosome in population:
fitness, _, _ = self.calculate_fitness(chromosome)
fitnesses.append(1 / fitness)
population = self.selection(population, fitnesses)
for j in range(len(population) // 2):
parent1 = population[2 * j]
parent2 = population[2 * j + 1]
child = self.crossover(parent1, parent2)
self.mutation(child)
population[2 * j] = child
population[2 * j + 1] = parent2
best_chromosome = max(population, key=lambda c: 1 / self.calculate_fitness(c)[0])
_, start_times, end_times = self.calculate_fitness(best_chromosome)
return start_times, end_times
# 示例数据
orders = [
Order(1, 10, 3, 0),
Order(2, 5, 2, 1),
Order(3, 20, 1, 2),
Order(4, 15, 4, 0),
Order(5, 7, 2, 1),
Order(6, 12, 3, 2),
Order(7, 18, 2, 0),
Order(8, 8, 1, 1),
Order(9, 13, 3, 2),
Order(10, 17, 2, 0),
]
production_lines = [5, 3, 4]
# 调用调度类求解最优方案
schedule = Schedule(orders, production_lines)
start_times, end_times = schedule.evolve()
# 输出结果
for i in range(len(orders)):
order = orders[i]
print('订单{}的开始作业时间为{},结束作业时间为{}'.format(order.order_id, start_times[i], end_times[i]))
```
在这个示例代码中,我们定义了一个包含10个订单和3条产线的调度问题,并使用遗传算法求解最优的生产调度方案。运行代码后,我们可以得到每个订单的开始作业时间和结束作业时间,从而得到一个完整的生产调度方案。
阅读全文
相关推荐
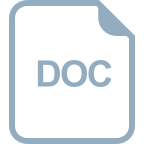
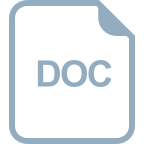
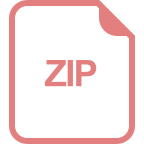
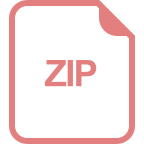
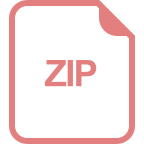
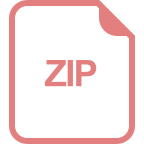
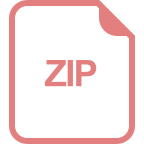
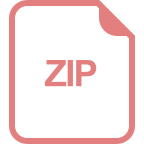
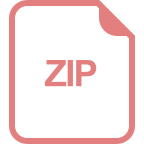
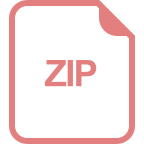
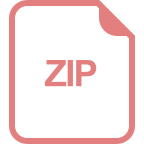
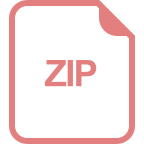
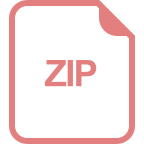
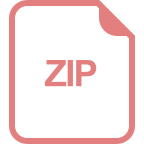
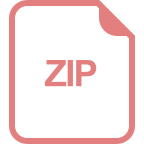
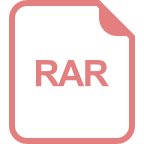
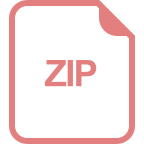
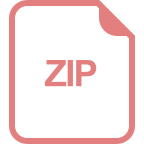