easypoi导出动态列及多级复杂表头java代码,要求不能定义实体类存储数据
时间: 2023-09-25 19:13:34 浏览: 102
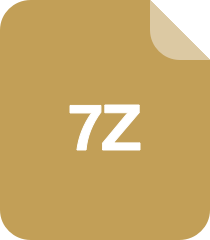
easypoi 动态列+详情导出示例
使用Easypoi导出动态列及多级复杂表头可以通过编写自定义的导出类来实现,以下是一个示例代码:
```java
public class CustomExportUtil {
/**
* 导出Excel
*
* @param title 标题
* @param headers 表头
* @param dataList 数据列表
* @param dateFormat 日期格式
* @param response HttpServletResponse对象
* @throws IOException
*/
public static void exportExcel(String title, List<ExcelHeader> headers, List<Map<String, Object>> dataList, String dateFormat, HttpServletResponse response) throws IOException {
// 创建Excel对象
Workbook workbook = new SXSSFWorkbook(1000);
// 创建Sheet对象
Sheet sheet = workbook.createSheet(title);
// 设置默认列宽
sheet.setDefaultColumnWidth(15);
// 创建第一行,设置表头
Row headerRow = sheet.createRow(0);
int colIndex = 0;
for (ExcelHeader header : headers) {
Cell cell = headerRow.createCell(colIndex++);
cell.setCellValue(header.getTitle());
// 设置合并单元格
if (header.getColSpan() > 1 || header.getRowSpan() > 1) {
CellRangeAddress rangeAddress = new CellRangeAddress(0, header.getRowSpan() - 1, colIndex - 1, colIndex + header.getColSpan() - 2);
sheet.addMergedRegion(rangeAddress);
}
}
// 填充数据
int rowIndex = 1;
for (Map<String, Object> data : dataList) {
Row row = sheet.createRow(rowIndex++);
colIndex = 0;
for (ExcelHeader header : headers) {
// 获取数据
Object value = data.get(header.getFieldName());
// 格式化日期
if (value instanceof Date) {
value = new SimpleDateFormat(dateFormat).format(value);
}
Cell cell = row.createCell(colIndex++);
cell.setCellValue(String.valueOf(value));
}
}
// 设置响应头
response.setContentType("application/octet-stream;charset=utf-8");
response.setHeader("Content-Disposition", "attachment;filename=" + new String((title + ".xlsx").getBytes("utf-8"), "iso8859-1"));
// 输出Excel文件
OutputStream outputStream = response.getOutputStream();
workbook.write(outputStream);
outputStream.flush();
outputStream.close();
}
}
```
其中,ExcelHeader类表示表头信息,包括标题、字段名、列合并数、行合并数等信息:
```java
public class ExcelHeader {
/**
* 标题
*/
private String title;
/**
* 字段名
*/
private String fieldName;
/**
* 列合并数
*/
private int colSpan;
/**
* 行合并数
*/
private int rowSpan;
public ExcelHeader(String title, String fieldName, int colSpan, int rowSpan) {
this.title = title;
this.fieldName = fieldName;
this.colSpan = colSpan;
this.rowSpan = rowSpan;
}
// getter/setter方法省略
}
```
使用示例:
```java
List<ExcelHeader> headers = new ArrayList<>();
headers.add(new ExcelHeader("姓名", "name", 1, 1));
headers.add(new ExcelHeader("年龄", "age", 1, 1));
headers.add(new ExcelHeader("联系方式", null, 2, 1));
headers.add(new ExcelHeader("电话", "phone", 1, 1));
headers.add(new ExcelHeader("邮箱", "email", 1, 1));
headers.add(new ExcelHeader("地址", "address", 1, 1));
headers.add(new ExcelHeader("省份", "province", 1, 1));
headers.add(new ExcelHeader("城市", "city", 1, 1));
headers.add(new ExcelHeader("区县", "county", 1, 1));
List<Map<String, Object>> dataList = new ArrayList<>();
Map<String, Object> data1 = new HashMap<>();
data1.put("name", "张三");
data1.put("age", 20);
data1.put("phone", "123456789");
data1.put("email", "zhangsan@example.com");
data1.put("address", "北京市朝阳区");
data1.put("province", "北京市");
data1.put("city", "北京市");
data1.put("county", "朝阳区");
dataList.add(data1);
CustomExportUtil.exportExcel("学生信息表", headers, dataList, "yyyy-MM-dd HH:mm:ss", response);
```
阅读全文
相关推荐
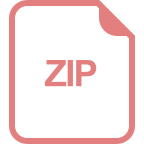
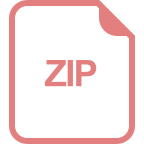










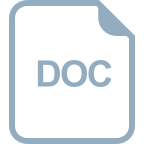
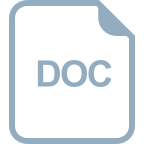
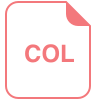

