设a为M行N列的二维数组,编程将a中的行和列交换, 交换后的数组仍然存放在a中,试建立一个类TDarray,完成以上操作。
时间: 2024-04-08 08:31:11 浏览: 107
下面是一个用C++编写的TDarray类的示例代码,该类可以实现将二维数组的行和列进行交换:
```cpp
#include <iostream>
#include <vector>
class TDarray {
private:
std::vector<std::vector<int>> array;
int rows;
int cols;
public:
TDarray(std::vector<std::vector<int>>& arr) {
array = arr;
rows = array.size();
cols = array[0].size();
}
void swapRowsAndCols() {
std::vector<std::vector<int>> newArray(cols, std::vector<int>(rows));
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
newArray[j][i] = array[i][j];
}
}
array = newArray;
std::cout << "行和列交换后的数组:" << std::endl;
printArray();
}
void printArray() {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
std::cout << array[i][j] << " ";
}
std::cout << std::endl;
}
}
};
int main() {
std::vector<std::vector<int>> arr = {{1, 2, 3},
{4, 5, 6},
{7, 8, 9}};
TDarray tdArray(arr);
std::cout << "原始数组:" << std::endl;
tdArray.printArray();
tdArray.swapRowsAndCols();
return 0;
}
```
在上述代码中,`TDarray`类具有一个私有成员变量`array`,用于存储二维数组。构造函数接受一个二维数组作为参数,将其存储在`array`中,并计算出行数`rows`和列数`cols`。`swapRowsAndCols`函数创建一个新的二维数组`newArray`,将原始数组的行和列进行交换,并将结果存储在`array`中。最后,通过`printArray`函数输出交换后的数组。
在`main`函数中,创建一个包含原始数组的`TDarray`对象,并调用`swapRowsAndCols`函数进行行和列的交换。然后,通过`printArray`函数输出交换后的数组。
请注意,该代码是一个简单的示例,假设输入的二维数组是正方形矩阵。如果需要处理不同大小的矩阵,可以根据实际需求进行修改和扩展。
阅读全文
相关推荐
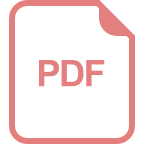
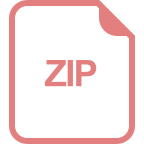




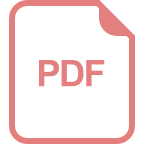
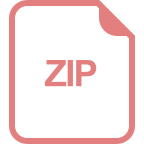
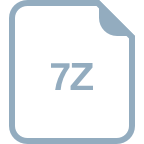
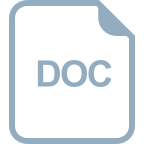
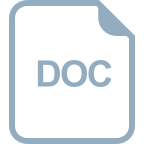
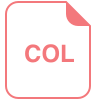




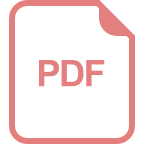
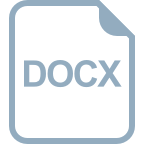
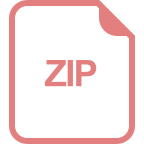