使用vue3循环生成li,给每个li添加各自的icon
时间: 2024-04-08 09:29:14 浏览: 21
你可以使用Vue 3的循环指令(v-for)来生成li,并使用动态绑定(class或style)来添加每个li的icon。以下是一个示例:
```html
<template>
<ul>
<li v-for="(item, index) in items" :key="index">
<i :class="item.icon"></i> {{ item.text }}
</li>
</ul>
</template>
<script>
export default {
data() {
return {
items: [
{ text: 'Item 1', icon: 'fa fa-star' },
{ text: 'Item 2', icon: 'fa fa-heart' },
{ text: 'Item 3', icon: 'fa fa-check' },
],
};
},
};
</script>
```
在上面的示例中,我们使用v-for指令循环遍历items数组,并为每个li元素绑定一个唯一的key。然后,我们使用动态绑定(:class)来为每个li添加对应的icon类名,可以使用Font Awesome等图标库提供的类名。最后,我们在每个li中显示item的文本。
请注意,上述示例中使用了Font Awesome图标库的类名,你可以根据需要自行替换为其他图标库或自定义样式。
相关问题
vue3侧边栏导航循环遍历icon
你可以使用`v-for`指令在Vue3中循环遍历侧边栏导航,同时使用第三方图标库(如Font Awesome)提供的icon。以下是一个示例代码:
```html
<template>
<div>
<ul>
<li v-for="(item, index) in navItems" :key="index">
<a :href="item.link">
<i :class="item.icon"></i> {{ item.title }}
</a>
</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
navItems: [
{
title: "Home",
link: "/",
icon: "fas fa-home" // Font Awesome提供的icon类名
},
{
title: "About",
link: "/about",
icon: "fas fa-info-circle"
},
{
title: "Contact",
link: "/contact",
icon: "fas fa-envelope"
},
// 循环遍历的数据可以自行添加或从后端API获取
]
};
}
};
</script>
```
在上面的代码中,`navItems`数组中存储了侧边栏导航的数据,包括每个导航项的标题、链接和图标类名。使用`v-for`指令在模板中循环遍历这些导航项,并将每个图标的类名绑定到`<i>`元素的`class`属性上,以显示相应的图标。
vue3生成随机盒子
要在Vue3中生成随机盒子,你可以使用grid布局和随机颜色来实现。首先,在你的template中,你可以使用ul和li标签来创建一个列表,将每个li标签作为一个盒子,然后使用v-for指令遍历一个包含随机颜色的数组。代码如下所示:
```html
<ul class="label_list">
<li class="label_item" v-for="(item, index) in skillTagArr" :style="{ backgroundColor: item.color }">
<i class="el-icon-share"></i>{{ item.tag }}
</li>
</ul>
```
接下来,在script标签中,你可以添加一个data属性来存储生成的随机颜色数组skillTagArr,并在mounted钩子函数中调用一个方法initTarget来初始化这个数组。在initTarget方法中,你可以将vuex仓库中的数据赋值给skillTagArr,并为每个对象添加一个color属性来存储随机生成的颜色。代码如下所示:
```javascript
import { mapState } from 'vuex'
export default {
data() {
return {
skillTagArr: [],
}
},
mounted() {
this.initTarget()
},
methods: {
initTarget() {
this.skillTagArr = this.personalInfo.tags
this.skillTagArr.forEach((item) => {
item.color = this.RandomColor()
})
},
RandomColor() {
let r, g, b
r = Math.floor(Math.random() * 256)
g = Math.floor(Math.random() * 256)
b = Math.floor(Math.random() * 256)
return 'rgb(' + r + ',' + g + ',' + b + ')'
},
},
computed: {
...mapState(['personalInfo']),
},
}
```
最后,你可以在style标签中定义grid-box容器以及盒子的样式。代码如下所示:
```css
<style scoped>
.grid-box {
display: grid;
grid-template-columns: auto auto auto auto auto auto;
grid-gap: 20px;
background-color: #2196F3;
padding: 10px;
box-sizing: border-box;
width: 700px;
height: 340px;
}
.grid-item {
background: #ccc;
}
.def-box {
background: #FFFFFF;
}
</style>
```
通过以上的代码,你可以在Vue3中生成一个带有随机颜色的盒子网格。每个盒子的颜色都是通过RandomColor方法随机生成的,并且通过v-for指令循环生成。
相关推荐
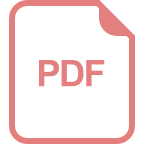
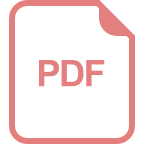












