Java根据IP获取城市
时间: 2024-02-04 11:12:20 浏览: 123
要根据IP获取城市,可以使用IP库来实现。以下是使用Java代码实现的示例:
首先需要下载一个IP库,例如QQWry.dat,然后使用如下代码进行查询:
```java
import com.github.jarod.qqwry.IPZone;
import com.github.jarod.qqwry.QQWry;
public class Main {
public static void main(String[] args) {
QQWry qqWry = new QQWry();
IPZone ipZone = qqWry.findIP("219.142.140.66");
System.out.println(ipZone.getMainInfo());
}
}
```
其中,`QQWry`是一个IP库解析工具,可以通过`findIP`方法查询IP地址所在的地区信息。`IPZone`则是查询结果的封装类,可以通过`getMainInfo`方法获取主要信息。
需要注意的是,这种方法只是根据IP地址来查询地区信息,可能存在一定的误差。
相关问题
麻烦帮我用Java代码实现通过IP获取城市名称(精确到县或者区)
如果你想通过IP地址来确定其对应的城市信息,你需要使用IP地址定位API,它可以通过IP地址来获取相关地理位置信息。
下面是一个使用第三方库来获取IP地址定位信息的简单示例:
```
import com.maxmind.geoip2.DatabaseReader;
import com.maxmind.geoip2.model.CityResponse;
import com.maxmind.geoip2.record.City;
import com.maxmind.geoip2.record.Country;
import com.maxmind.geoip2.record.Location;
import com.maxmind.geoip2.record.Postal;
import com.maxmind.geoip2.record.Subdivision;
import java.io.File;
import java.net.InetAddress;
public class GeoLocationExample {
public static void main(String[] args) throws Exception {
// A File object pointing to your GeoIP2 or GeoLite2 database
File database = new File("/path/to/GeoLite2-City.mmdb");
// This creates the DatabaseReader object, which should be reused across
// lookups.
DatabaseReader reader = new DatabaseReader.Builder(database).build();
InetAddress ipAddress = InetAddress.getByName("8.8.8.8");
// Replace "city" with the appropriate method for your database, e.g.,
// "country".
CityResponse response = reader.city(ipAddress);
Country country = response.getCountry();
System.out.println(country.getName()); // 'United States'
Subdivision subdivision = response.getMostSpecificSubdivision();
System.out.println(subdivision.getName()); // 'Minnesota'
System.out.println(subdivision.getIsoCode()); // 'MN'
City city = response.getCity();
System.out.println(city.getName()); // 'Minneapolis'
Postal postal = response.getPostal();
System.out.println(postal.getCode()); // '55455'
Location location = response.getLocation();
System.out.println(location.getLatitude()); // 44.9733
System.out.println(location.getLongitude()); // -93.2323
}
}
```
上面的代码使用了MaxMind GeoIP2 Java API,该API是一个商业解决方案,需要购买合适的数据库才能使用。你也可以尝试使用
java 获取ip归属地
在 Java 中,我们可以使用第三方库来获取 IP 的归属地信息。其中一个常用的库是 GeoIP2,它基于 MaxMind 的 GeoIP2 数据库。
首先,需要将 GeoIP2 库添加到项目中。可以在 Maven 或 Gradle 构建脚本中添加相应的依赖项。
然后,我们可以使用 GeoIP2 提供的 API 来查询 IP 的归属地。以下是一个示例代码:
```java
import com.maxmind.geoip2.DatabaseReader;
import com.maxmind.geoip2.model.CityResponse;
import com.maxmind.geoip2.record.Country;
import java.io.File;
import java.io.IOException;
import java.net.InetAddress;
public class IPUtils {
public static String getIPCountry(String ip) {
try {
File database = new File("GeoIP2-City.mmdb");
DatabaseReader reader = new DatabaseReader.Builder(database).build();
InetAddress ipAddress = InetAddress.getByName(ip);
CityResponse response = reader.city(ipAddress);
Country country = response.getCountry();
return country.getName();
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
public static void main(String[] args) {
String ip = "123.456.789.0";
String country = getIPCountry(ip);
System.out.println("IP " + ip + " 的归属地是:" + country);
}
}
```
在上述示例中,我们通过 `getIPCountry` 方法传入一个 IP 地址,并在 `main` 方法中调用该方法来获取该 IP 的归属地信息。具体的归属地信息包括国家、地区、城市等,可以根据需要进行扩展和处理。
需要注意的是,在运行代码之前,我们需要下载并导入 GeoIP2 数据库文件 `GeoIP2-City.mmdb`,该文件包含了 IP 地址和归属地信息的映射关系。可以从 MaxMind 的官网或其他数据源获取该文件。
这样,我们就可以通过 Java 来获取 IP 的归属地信息了。
阅读全文
相关推荐
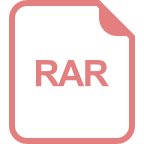

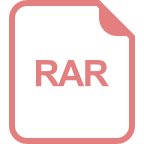






