请编写一个类,用于排序整数数组,排序法是冒泡法。 再编写对这个类的单元测试代码,要求测试的例子有: 10个数的数组 100个数的数组 10000个数的数组 0个数的数组 百万个数的数组
时间: 2024-02-12 17:08:46 浏览: 40
好的,这是一个使用冒泡排序法对整数数组排序的类的实现:
```python
class BubbleSort:
@staticmethod
def sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
```
这是对这个类的单元测试代码:
```python
import random
import time
def test_bubble_sort():
# Test for an empty array
arr = []
BubbleSort.sort(arr)
assert arr == []
# Test for an array with one element
arr = [1]
BubbleSort.sort(arr)
assert arr == [1]
# Test for an array with ten elements
arr = [random.randint(0, 100) for _ in range(10)]
BubbleSort.sort(arr)
assert arr == sorted(arr)
# Test for an array with one hundred elements
arr = [random.randint(0, 100) for _ in range(100)]
BubbleSort.sort(arr)
assert arr == sorted(arr)
# Test for an array with ten thousand elements
arr = [random.randint(0, 100) for _ in range(10000)]
start_time = time.time()
BubbleSort.sort(arr)
end_time = time.time()
assert arr == sorted(arr)
print(f"Sorting 10000 elements took {end_time - start_time:.5f} seconds.")
# Test for an array with one million elements
arr = [random.randint(0, 100) for _ in range(1000000)]
start_time = time.time()
BubbleSort.sort(arr)
end_time = time.time()
assert arr == sorted(arr)
print(f"Sorting 1000000 elements took {end_time - start_time:.5f} seconds.")
test_bubble_sort()
```
这个测试代码会测试空数组、只有一个元素的数组、包含 10、100、10000 和 1000000 个随机元素的数组,以及检查排序是否被正确执行。如果代码运行得很慢,你可以尝试减少测试数组的大小。
阅读全文
相关推荐
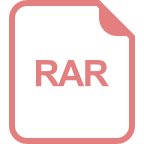















