把Java所有的关键字找出,并给出代码实例。
时间: 2023-04-07 11:01:54 浏览: 118
Java所有的关键字有:abstract、assert、boolean、break、byte、case、catch、char、class、const、continue、default、do、double、else、enum、extends、false、final、finally、float、for、goto、if、implements、import、instanceof、int、interface、long、native、new、null、package、private、protected、public、return、short、static、strictfp、super、switch、synchronized、this、throw、throws、transient、true、try、void、volatile、while。
以下是一些代码实例:
1. abstract关键字:
abstract class Animal {
public abstract void makeSound();
}
2. boolean关键字:
boolean isRaining = true;
3. break关键字:
for (int i = 0; i < 10; i++) {
if (i == 5) {
break;
}
System.out.println(i);
}
4. case关键字:
switch (dayOfWeek) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
// ...
}
5. catch关键字:
try {
// some code that may throw an exception
} catch (Exception e) {
// handle the exception
}
6. class关键字:
class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
7. continue关键字:
for (int i = 0; i < 10; i++) {
if (i == 5) {
continue;
}
System.out.println(i);
}
8. default关键字:
switch (dayOfWeek) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
// ...
default:
System.out.println("Invalid day of week");
}
9. do关键字:
int i = 0;
do {
System.out.println(i);
i++;
} while (i < 10);
10. double关键字:
double pi = 3.14159;
11. else关键字:
if (isRaining) {
System.out.println("Bring an umbrella");
} else {
System.out.println("Leave the umbrella at home");
}
12. enum关键字:
enum DayOfWeek {
MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY
}
13. extends关键字:
class Dog extends Animal {
public void makeSound() {
System.out.println("Woof!");
}
}
14. false关键字:
boolean isRaining = false;
15. final关键字:
final int MAX_VALUE = 100;
16. finally关键字:
try {
// some code that may throw an exception
} catch (Exception e) {
// handle the exception
} finally {
// code that will always execute, regardless of whether an exception was thrown or not
}
17. float关键字:
float pi = 3.14159f;
18. for关键字:
for (int i = 0; i < 10; i++) {
System.out.println(i);
}
19. if关键字:
if (isRaining) {
System.out.println("Bring an umbrella");
}
20. implements关键字:
class Dog implements Animal {
public void makeSound() {
System.out.println("Woof!");
}
}
21. import关键字:
import java.util.ArrayList;
22. instanceof关键字:
if (animal instanceof Dog) {
Dog dog = (Dog) animal;
dog.makeSound();
}
23. int关键字:
int age = 30;
24. interface关键字:
interface Animal {
void makeSound();
}
25. long关键字:
long population = 1000000000L;
26. new关键字:
Person person = new Person("Alice", 25);
27. null关键字:
String name = null;
28. package关键字:
package com.example.myapp;
29. private关键字:
private String name;
30. protected关键字:
protected int age;
31. public关键字:
public void setName(String name) {
this.name = name;
}
32. return关键字:
public int add(int a, int b) {
return a + b;
}
33. short关键字:
short temperature = 20;
34. static关键字:
static int count = 0;
35. super关键字:
class Dog extends Animal {
public void makeSound() {
super.makeSound();
System.out.println("Woof!");
}
}
36. switch关键字:
switch (dayOfWeek) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
// ...
}
37. synchronized关键字:
synchronized (lock) {
// code that needs to be executed atomically
}
38. this关键字:
public String getName() {
return this.name;
}
39. throw关键字:
if (age < 0) {
throw new IllegalArgumentException("Age cannot be negative");
}
40. throws关键字:
public void someMethod() throws IOException {
// code that may throw an IOException
}
41. try关键字:
try {
// some code that may throw an exception
} catch (Exception e) {
// handle the exception
}
42. void关键字:
public void someMethod() {
// code that does not return anything
}
43. while关键字:
int i = 0;
while (i < 10) {
System.out.println(i);
i++;
}
阅读全文
相关推荐




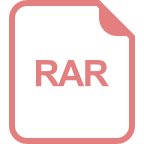
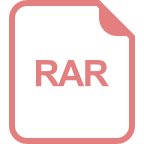


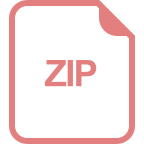

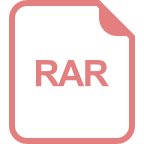



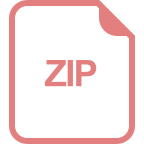
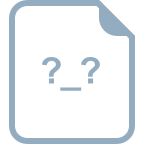



