pytorch写对抗神经网络学习波士顿房价
时间: 2023-07-18 16:12:02 浏览: 113
下面是使用PyTorch实现对抗神经网络学习波士顿房价的示例代码:
```python
import torch
import torch.nn as nn
import torch.optim as optim
from sklearn.datasets import load_boston
from sklearn.preprocessing import StandardScaler
# 加载数据集并进行预处理
boston = load_boston()
scaler = StandardScaler()
X = scaler.fit_transform(boston.data)
y = scaler.fit_transform(boston.target.reshape(-1, 1))
X = torch.from_numpy(X.astype('float32'))
y = torch.from_numpy(y.astype('float32'))
# 定义生成器模型
class Generator(nn.Module):
def __init__(self, input_size, output_size, hidden_size):
super(Generator, self).__init__()
self.net = nn.Sequential(
nn.Linear(input_size, hidden_size),
nn.ReLU(),
nn.Linear(hidden_size, hidden_size),
nn.ReLU(),
nn.Linear(hidden_size, output_size)
)
def forward(self, x):
return self.net(x)
# 定义判别器模型
class Discriminator(nn.Module):
def __init__(self, input_size, hidden_size):
super(Discriminator, self).__init__()
self.net = nn.Sequential(
nn.Linear(input_size, hidden_size),
nn.ReLU(),
nn.Linear(hidden_size, hidden_size),
nn.ReLU(),
nn.Linear(hidden_size, 1),
nn.Sigmoid()
)
def forward(self, x):
return self.net(x)
# 定义模型参数
input_size = X.shape[1]
hidden_size = 32
output_size = y.shape[1]
lr = 0.001
batch_size = 64
num_epochs = 100
# 初始化模型和优化器
G = Generator(input_size, output_size, hidden_size)
D = Discriminator(input_size + output_size, hidden_size)
G_optimizer = optim.Adam(G.parameters(), lr)
D_optimizer = optim.Adam(D.parameters(), lr)
criterion = nn.BCELoss()
# 定义训练函数
def train():
for epoch in range(num_epochs):
for i in range(0, X.shape[0], batch_size):
# 训练判别器
X_batch = X[i:i+batch_size]
y_batch = y[i:i+batch_size]
noise = torch.randn(batch_size, input_size)
fake_data = G(noise)
D_real = D(torch.cat([X_batch, y_batch], dim=1))
D_fake = D(torch.cat([noise, fake_data], dim=1))
D_real_loss = criterion(D_real, torch.ones_like(D_real))
D_fake_loss = criterion(D_fake, torch.zeros_like(D_fake))
D_loss = D_real_loss + D_fake_loss
D_optimizer.zero_grad()
D_loss.backward()
D_optimizer.step()
# 训练生成器
noise = torch.randn(batch_size, input_size)
fake_data = G(noise)
D_fake = D(torch.cat([noise, fake_data], dim=1))
G_loss = criterion(D_fake, torch.ones_like(D_fake))
G_optimizer.zero_grad()
G_loss.backward()
G_optimizer.step()
# 打印损失
print('Epoch [{}/{}], D_loss: {:.4f}, G_loss: {:.4f}'.format(epoch+1, num_epochs, D_loss.item(), G_loss.item()))
# 训练模型
train()
# 生成新的房价数据
noise = torch.randn(100, input_size)
fake_data = G(noise)
fake_data = scaler.inverse_transform(fake_data.detach().numpy())
print('Generated Data: \n', fake_data)
```
这段代码使用对抗神经网络来学习波士顿房价数据集。首先,加载数据集并进行预处理,然后定义生成器和判别器模型,以及相关的训练参数和优化器。在训练过程中,先训练判别器,再训练生成器,通过对抗训练来提高生成器的生成能力。最后,使用生成器来生成新的房价数据。
阅读全文
相关推荐
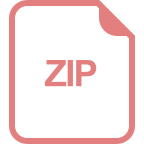
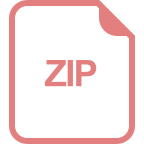


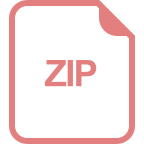
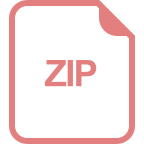
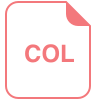
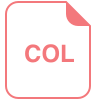
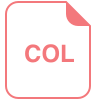
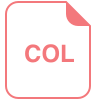
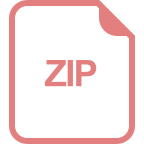
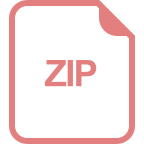
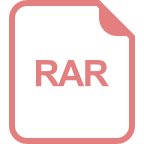
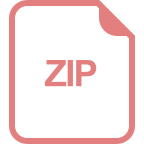
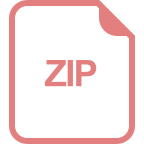