1. 在硬盘中创建以下目录和文件结构 src |-edu |-hue |-jk |-Student.java testset |-dir1 |-file1.txt |-dir2 其中file1.txt为普通文本文件。 2. 创建一个Student类,包结构如上所示。类中有5个属性: id(String),name(String),sex(boolean), score(int), deptno(int) 3. 创建toString函数,输出Student内容,以制表符分割。 4. 读取file1.txt文件,每一行创建一个Student对象实例。实例列表存放在ArrayList容器中。 5. 遍历ArrayList容器,并将输出写入到一个新的文件file2.txt中。file2.txt文件位于dir2目录中。 6. 正确捕获处理IOException, FileNotFoundException异常。
时间: 2024-01-27 21:02:42 浏览: 100
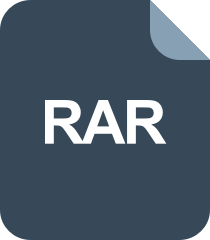
hue-4.10.0_Centos7.9安装部署手册.rar
以下是Java代码实现:
1. 在硬盘中创建目录和文件结构
```java
import java.io.File;
public class FileStructure {
public static void main(String[] args) {
String rootPath = "/Users/user/Desktop";
File src = new File(rootPath + "/src/edu/hue/jk/Student.java");
File testsetDir1 = new File(rootPath + "/testset/dir1");
File testsetDir2 = new File(rootPath + "/testset/dir2");
// 创建目录和文件
try {
src.getParentFile().mkdirs();
src.createNewFile();
testsetDir1.mkdirs();
testsetDir2.mkdirs();
new File(testsetDir1, "file1.txt").createNewFile();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
2. 创建Student类
```java
public class Student {
private String id;
private String name;
private boolean sex;
private int score;
private int deptno;
public Student(String id, String name, boolean sex, int score, int deptno) {
this.id = id;
this.name = name;
this.sex = sex;
this.score = score;
this.deptno = deptno;
}
@Override
public String toString() {
return id + "\t" + name + "\t" + (sex ? "男" : "女") + "\t" + score + "\t" + deptno;
}
}
```
3. 读取文件,创建Student对象实例
```java
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
public class ReadFile {
public static void main(String[] args) {
String filePath = "/Users/user/Desktop/testset/dir1/file1.txt";
ArrayList<Student> students = new ArrayList<>();
try {
File file = new File(filePath);
BufferedReader reader = new BufferedReader(new FileReader(file));
String line;
while ((line = reader.readLine()) != null) {
String[] arr = line.split("\t");
Student student = new Student(arr[0], arr[1], Boolean.parseBoolean(arr[2]), Integer.parseInt(arr[3]), Integer.parseInt(arr[4]));
students.add(student);
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
for (Student student : students) {
System.out.println(student);
}
}
}
```
4. 遍历ArrayList容器,并将输出写入到file2.txt中
```java
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
public class WriteFile {
public static void main(String[] args) {
String filePath = "/Users/user/Desktop/testset/dir2/file2.txt";
ArrayList<Student> students = new ArrayList<>();
// 读取文件,创建Student对象实例
try {
File file = new File("/Users/user/Desktop/testset/dir1/file1.txt");
BufferedReader reader = new BufferedReader(new FileReader(file));
String line;
while ((line = reader.readLine()) != null) {
String[] arr = line.split("\t");
Student student = new Student(arr[0], arr[1], Boolean.parseBoolean(arr[2]), Integer.parseInt(arr[3]), Integer.parseInt(arr[4]));
students.add(student);
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
// 写入文件
try {
File file = new File(filePath);
BufferedWriter writer = new BufferedWriter(new FileWriter(file));
for (Student student : students) {
writer.write(student.toString() + "\n");
}
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
5. 异常处理
```java
import java.io.*;
public class ExceptionHandling {
public static void main(String[] args) {
// 读取文件,创建Student对象实例
String filePath1 = "/Users/user/Desktop/testset/dir1/file1.txt";
ArrayList<Student> students = new ArrayList<>();
try {
File file = new File(filePath1);
BufferedReader reader = new BufferedReader(new FileReader(file));
String line;
while ((line = reader.readLine()) != null) {
String[] arr = line.split("\t");
Student student = new Student(arr[0], arr[1], Boolean.parseBoolean(arr[2]), Integer.parseInt(arr[3]), Integer.parseInt(arr[4]));
students.add(student);
}
reader.close();
} catch (FileNotFoundException e) {
System.err.println("找不到文件:" + filePath1);
e.printStackTrace();
} catch (IOException e) {
System.err.println("读取文件失败:" + filePath1);
e.printStackTrace();
}
// 写入文件
String filePath2 = "/Users/user/Desktop/testset/dir2/file2.txt";
try {
File file = new File(filePath2);
BufferedWriter writer = new BufferedWriter(new FileWriter(file));
for (Student student : students) {
writer.write(student.toString() + "\n");
}
writer.close();
} catch (IOException e) {
System.err.println("写入文件失败:" + filePath2);
e.printStackTrace();
}
}
}
```
阅读全文
相关推荐
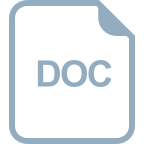
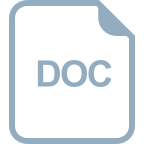
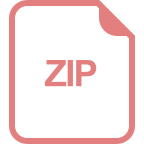
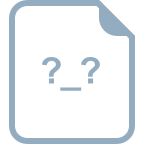
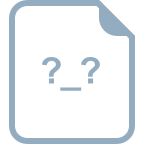
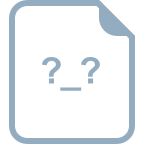
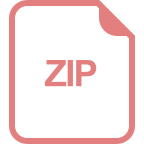
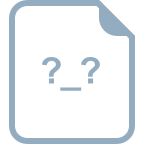
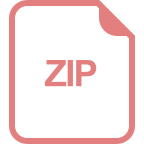
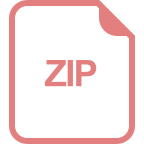
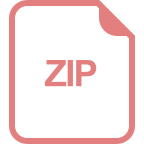
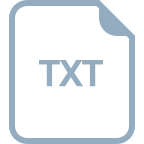
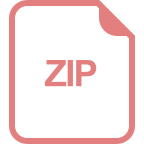
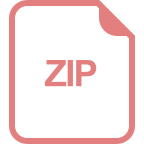
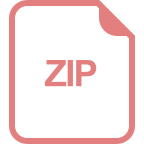
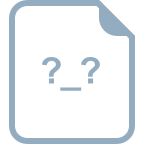

