pytorch用两个网络模型解决poisson方程界面问题代码实现
时间: 2024-02-20 14:01:17 浏览: 82
下面是一个简单的代码实现,使用两个神经网络模型来解决Poisson方程界面问题。在这个例子中,我们使用的是均方误差损失函数和Adam优化器。
```python
import torch
import torch.nn as nn
import torch.optim as optim
# 定义Poisson方程网络模型
class PoissonNet(nn.Module):
def __init__(self):
super(PoissonNet, self).__init__()
self.fc1 = nn.Linear(3, 10)
self.fc2 = nn.Linear(10, 1)
def forward(self, x):
x = torch.sigmoid(self.fc1(x))
x = self.fc2(x)
return x
# 定义界面条件网络模型
class InterfaceNet(nn.Module):
def __init__(self):
super(InterfaceNet, self).__init__()
self.fc1 = nn.Linear(3, 10)
self.fc2 = nn.Linear(10, 1)
def forward(self, x):
x = torch.sigmoid(self.fc1(x))
x = self.fc2(x)
return x
# 定义Poisson方程的边界条件
def boundary(x):
return torch.sin(x)
# 定义Poisson方程的初始条件
def initial(x):
return torch.zeros_like(x)
# 定义Poisson方程的真实解
def true_solution(x):
return torch.sin(x)
# 定义训练数据
train_data = torch.randn(100, 3)
# 初始化Poisson方程模型和界面条件模型
poisson_model = PoissonNet()
interface_model = InterfaceNet()
# 定义优化器和损失函数
poisson_optimizer = optim.Adam(poisson_model.parameters())
interface_optimizer = optim.Adam(interface_model.parameters())
criterion = nn.MSELoss()
# 训练模型
for epoch in range(1000):
# 使用Poisson方程模型求解
x = train_data.clone().detach().requires_grad_(True)
y = poisson_model(x)
dydx = torch.autograd.grad(y, x, grad_outputs=torch.ones_like(y), create_graph=True)[0]
d2ydx2 = torch.autograd.grad(dydx, x, grad_outputs=torch.ones_like(dydx), create_graph=True)[0]
poisson_loss = criterion(d2ydx2, -1 * boundary(x))
poisson_optimizer.zero_grad()
poisson_loss.backward()
poisson_optimizer.step()
# 使用界面条件模型求解
x = train_data.clone().detach().requires_grad_(True)
y = interface_model(x)
full_solution = y + initial(x)
interface_loss = criterion(full_solution, true_solution(x))
interface_optimizer.zero_grad()
interface_loss.backward()
interface_optimizer.step()
if epoch % 100 == 0:
print(f"Epoch {epoch}: Poisson loss {poisson_loss.item()}, Interface loss {interface_loss.item()}")
```
在这个例子中,我们使用了随机生成的数据来训练模型。在实际应用中,需要根据特定问题的边界条件和初始条件来生成训练数据。
阅读全文
相关推荐
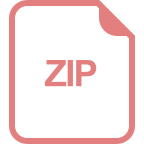
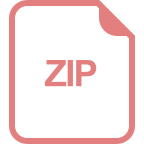
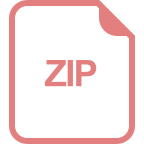
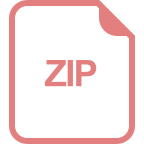
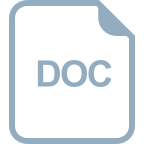
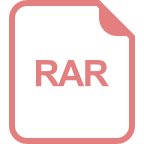
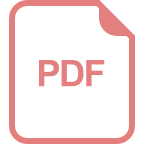
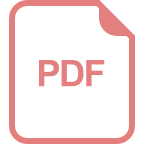
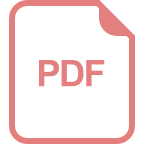
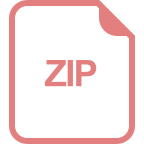
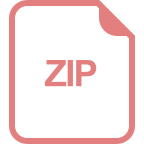
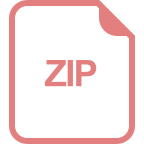